NumPy: Create a 5x5 matrix with row values ranging from 0 to 4
NumPy: Array Object Exercise-64 with Solution
Write a NumPy program to create a 5x5 matrix with row values ranging from 0 to 4.
Pictorial Presentation:
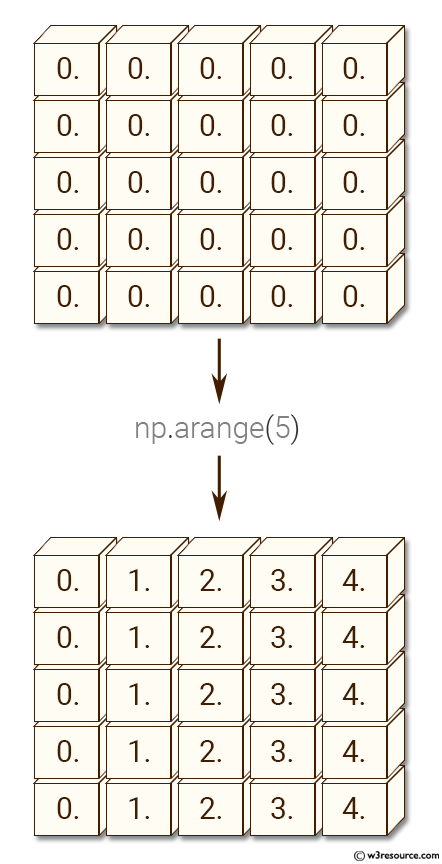
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 5x5 NumPy array 'x' filled with zeros
x = np.zeros((5,5))
# Displaying the original 5x5 array 'x' filled with zeros
print("Original array:")
print(x)
# Adding row values ranging from 0 to 4 to each row of the array 'x'
# The values range from 0 to 4 due to np.arange(5)
x += np.arange(5)
# Displaying the array 'x' after adding row values
print("Row values ranging from 0 to 4.")
print(x)
Sample Output:
Original array: [[ 0. 0. 0. 0. 0.] [ 0. 0. 0. 0. 0.] [ 0. 0. 0. 0. 0.] [ 0. 0. 0. 0. 0.] [ 0. 0. 0. 0. 0.]] Row values ranging from 0 to 4. [[ 0. 1. 2. 3. 4.] [ 0. 1. 2. 3. 4.] [ 0. 1. 2. 3. 4.] [ 0. 1. 2. 3. 4.] [ 0. 1. 2. 3. 4.]]
Explanation:
‘x = np.zeros((5,5))’ creates a 5x5 array filled with zeros and stores in the variable ‘x’.
x += np.arange(5): This line adds the elements of the 1D array np.arange(5) to each row of the 2D array ‘x’. The np.arange(5) function creates a 1D array of elements from 0 to 4. Due to broadcasting rules, the 1D array is automatically expanded to match the shape of the 2D array x, and the elements are added element-wise.
Python-Numpy Code Editor:
Previous: Write a NumPy program to get the number of nonzero elements in an array.
Next: Write a NumPy program to test if specified values are present in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/python-numpy-exercise-64.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics