NumPy: Test whether specified values are present in an array
Check Values Present in Array
Write a NumPy program to test if specified values are present in an array.
Pictorial Presentation:
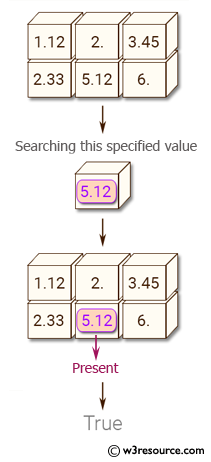
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 2x3 NumPy array 'x' with specific float values
x = np.array([[1.12, 2.0, 3.45], [2.33, 5.12, 6.0]], float)
# Displaying the original array 'x'
print("Original array:")
print(x)
# Checking if 2 is present in the array 'x'
print(2 in x)
# Checking if 0 is present in the array 'x'
print(0 in x)
# Checking if 6 is present in the array 'x'
print(6 in x)
# Checking if 2.3 is present in the array 'x'
print(2.3 in x)
# Checking if 5.12 is present in the array 'x'
print(5.12 in x)
Sample Output:
Original array: [[ 1.12 2. 3.45] [ 2.33 5.12 6. ]] True False True False True
Explanation:
In the above exercise -
‘x = np.array([[1.12, 2.0, 3.45], [2.33, 5.12, 6.0]], float)’ creates a 2D NumPy array x with the specified floating-point values.
The following statements use the in operator to check if the specified values are present in the array x:
- print(2 in x): Checks if 2 is present in the array. Prints True because 2.0 is present.
- print(0 in x): Checks if 0 is present in the array. Prints False because 0 is not present.
- print(6 in x): Checks if 6 is present in the array. Prints True because 6.0 is present.
- print(2.3 in x): Checks if 2.3 is present in the array. Prints False because 2.3 is not present.
- print(5.12 in x): Checks if 5.12 is present in the array. Prints True because 5.12 is present.
For more Practice: Solve these Related Problems:
- Write a NumPy program to test if specified values exist in an array using np.isin.
- Create a function that takes an array and a list of target values, returning a boolean array for membership.
- Verify the presence of values by comparing the output of np.in1d with a custom iteration method.
- Use logical operations to check if multiple specific values are simultaneously present in a given array.
Go to:
PREV : 5x5 Matrix with Row Values 0–4
NEXT : Vector of Size 10 (0–1 Excluded)
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.