NumPy: Sum of all the multiples of 3 or 5 below 100
Sum Multiples of 3 or 5 Below 100
Write a NumPy program (using numpy) to sum all the multiples of 3 or 5 below 100.
Pictorial Presentation:
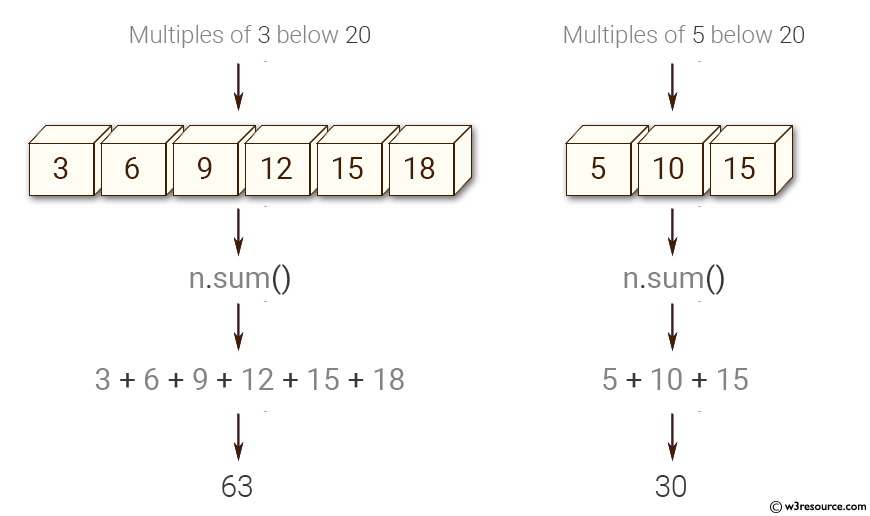
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating an array 'x' using NumPy's arange function,
# generating values from 1 to 99 (inclusive) since the end value is exclusive
x = np.arange(1, 100)
# Finding numbers in the array 'x' that are multiples of 3 or 5
n = x[(x % 3 == 0) | (x % 5 == 0)]
# Printing the first 1000 elements of the array 'n'
print(n[:1000])
# Printing the sum of the numbers in the array 'n'
print(n.sum())
Sample Output:
[ 3 5 6 9 10 12 15 18 20 21 24 25 27 30 33 35 36 39 40 42 45 48 50 5 1 54 55 57 60 63 65 66 69 70 72 75 78 80 81 84 85 87 90 93 95 96 99] 2318
Explanation:
In the above exercise –
‘x = np.arange(1, 100)’ creates a 1D NumPy array with integers from 1 to 99 and stores it in the variable ‘x’.
n = x[(x % 3 == 0) | (x % 5 == 0)]: This line creates a boolean mask for elements in ‘x’ that are divisible by 3 or 5. The % operator computes the remainder of the division, and == 0 checks if the remainder is 0. The | operator is a boolean OR, which combines the two conditions. The resulting mask is then used to index ‘x’, creating a new array ‘n’ containing the numbers divisible by either 3 or 5.
print(n[:1000]): This part prints the first 1000 elements of the n array. Since there are fewer than 1000 elements in n, this will print all elements in the array.
print(n.sum()): This part calculates the sum of all elements in the n array and prints the result.
For more Practice: Solve these Related Problems:
- Write a NumPy program to identify and sum all multiples of 3 or 5 below 100 using vectorized operations.
- Create a function that filters multiples of 3 or 5 from an array and computes their total sum.
- Compare the performance of summing multiples using np.where and np.sum versus a loop-based approach.
- Test the solution by verifying both the list of multiples and the computed sum against expected values.
Go to:
PREV : Make Array Read-Only
NEXT : Create Array with 10^3 Elements
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.