NumPy: Create a 5x5x5 cube of 1's
NumPy: Array Object Exercise-72 with Solution
Write a NumPy program to create a 5x5x5 cube of 1's.
Pictorial Presentation:
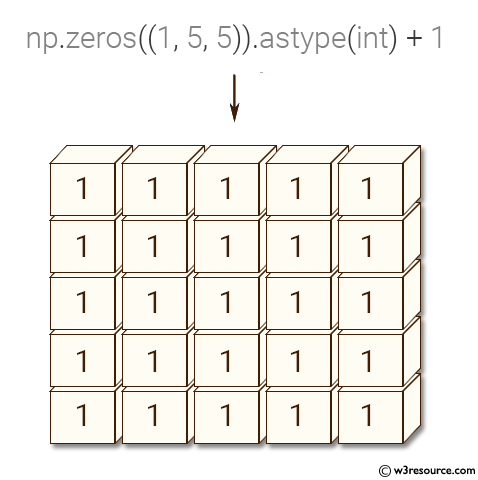
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 3-dimensional array filled with zeros of size 5x5x5 and converting it to integers
# Adding 1 to each element of the array using the 'astype' method
x = np.zeros((5, 5, 5)).astype(int) + 1
# Printing the resulting array 'x' filled with the value 1
print(x)
Sample Output:
[[[1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1]] [[1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1]] [[1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1]] [[1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1]] [[1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1] [1 1 1 1 1]]]
Explanation:
The above code creates a 5x5x5 NumPy array filled with ones (integer data type).
np.zeros((5, 5, 5)): This function call creates a 3D NumPy array with dimensions 5x5x5, filled with zeros. By default, the elements are of float data type.
.astype(int): This method converts the data type of the elements in the array from float to integer.
+ 1: This operation adds 1 to each element in the integer array. Since the array was initially filled with zeros, adding 1 to each element results in an array filled with ones.
Finally print(x) function prints the 5x5x5 integer array filled with ones.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create and display every element of an numpy array in Fortran order.
Next: Write a NumPy program to create an array of (3, 4) shape, multiply every element value by 3 and display the new array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/python-numpy-exercise-72.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics