NumPy: Create an array of (3, 4) shape, multiply every element value by 3 and display the new array
NumPy: Array Object Exercise-73 with Solution
Write a NumPy program to create an array of (3, 4) shapes, multiply every element value by 3 and display the result array.
Pictorial Presentation:
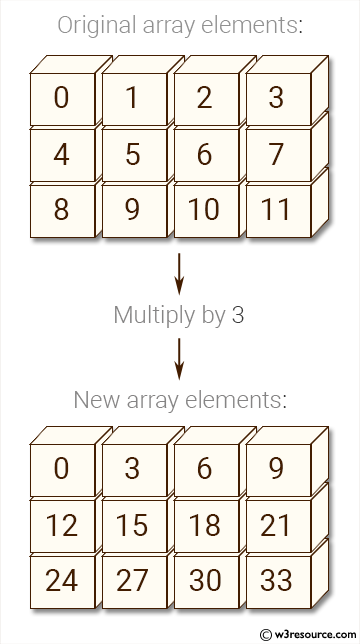
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 1-dimensional array 'x' with values from 0 to 11 and reshaping it into a 3x4 array
x = np.arange(12).reshape(3, 4)
# Printing a message indicating the original array elements will be shown
print("Original array elements:")
# Printing the original array 'x' with its elements
print(x)
# Using a loop with np.nditer to iterate through each element in the array 'x'
# Setting the elements to 3 times their current value with op_flags set to 'readwrite'
for a in np.nditer(x, op_flags=['readwrite']):
a[...] = 3 * a # Multiplying each element by 3
# Printing a message indicating the new array elements after multiplication
print("New array elements:")
# Printing the array 'x' after modifying its elements (each element multiplied by 3)
print(x)
Sample Output:
Original array elements: [[ 0 1 2 3] [ 4 5 6 7] [ 8 9 10 11]] New array elements: [[ 0 3 6 9] [12 15 18 21] [24 27 30 33]]
Explanation:
In the above code:
- np.arange(12): This function call creates a 1D NumPy array with integers from 0 to 11.
- reshape(3, 4): This method reshapes the 1D array into a 3x4 2D array.
- for a in np.nditer(x, op_flags=['readwrite']):: This part initializes a loop using np.nditer to iterate over each element of the 2D array x. The op_flags=['readwrite'] argument allows the iterator to read and modify the elements in the original array.
- a[...] = 3 * a: Inside the loop, this line multiplies each element of the array by 3 and assigns the result back to the original location in the array.
- Finally print(x) prints the modified 2D array.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create a 5x5x5 cube of 1's.
Next: Write a NumPy program to combine a one and a two dimensional array together and display their elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/numpy/python-numpy-exercise-73.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics