Read PNG image data into NumPy array using Python
NumPy: I/O Operations Exercise-18 with Solution
Write a NumPy program that read image data from a PNG file into a NumPy array using an image processing library like PIL or opencv.
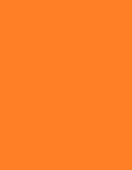
Sample Solution:
Python Code:
from PIL import Image
import numpy as np
# Define the path to the PNG file
image_path = 'image.png'
# Open the image file using PIL
image = Image.open(image_path)
# Convert the image to a NumPy array
image_array = np.array(image)
# Print the shape of the image array to verify
print('Image array shape:', image_array.shape)
# Display the image array (optional, requires matplotlib)
import matplotlib.pyplot as plt
plt.imshow(image_array)
plt.show()
Output:
Image array shape: (170, 132, 3)
Explanation:
- Import Libraries:
- Imported Image from the PIL library for image processing.
- Imported numpy as np for handling arrays.
- Define Image Path:
- Set the path to the PNG file as image_path.
- Open Image File:
- Used Image.open to open the image file specified by image_path.
- Convert Image to NumPy Array:
- Converted the image object to a NumPy array using np.array.
- Print Image Array Shape:
- Printed the shape of the NumPy array to verify the conversion.
- Display Image Array:
- Optionally displayed the image using matplotlib.pyplot to visualize the array data.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics