Loading a CSV file into a Pandas DataFrame with Python
Load a CSV file into a Pandas DataFrame.
test.csv:
col1,col2,col3 20,40,60 30,50,80 40,60,100
Sample Solution:
Python Code:
import pandas as pd
# Replace 'your_file.csv' with the actual path to your CSV file
file_path = 'test.csv'
# Load CSV file into a Pandas DataFrame
df = pd.read_csv(file_path)
# Display the DataFrame
print(df)
Output:
col1 col2 col3 0 20 40 60 1 30 50 80 2 40 60 100
Explanation:
- importing Pandas Library:
import pandas as pd - Import the Pandas library and alias it as "pd" for convenience. This is a common convention in the Python data science community. - Specifying the File Path:
file_path = 'test.csv' - Replace 'test.csv' with the actual path to your CSV file. This variable holds the path or URL of the CSV file that you want to load into the DataFrame. - Loading CSV File into DataFrame:
df = pd.read_csv(file_path) - The "read_csv()" function reads the CSV file and create a Pandas DataFrame (df). It automatically detects the delimiter (comma, semicolon, etc.) in the CSV file. If your CSV file has a different delimiter, you can specify it using the 'sep' parameter. For example,
df = pd.read_csv(file_path, sep=';') # Replace ';' with your actual delimiter. - Displaying the DataFrame:
print(df) - This line prints the DataFrame contents to the console. You'll see the data, column names, and other information about the DataFrame.
Flowchart:
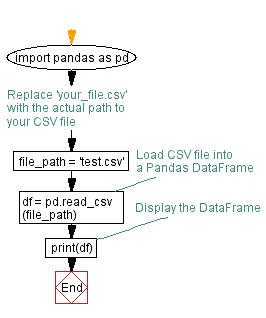
Python Code Editor:
Previous: Pandas Numpy Exercise Home.
Next: Generating a Pandas DataFrame from a NumPy array with custom column names in Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.