Performing element-wise addition in NumPy arrays
Perform element-wise addition on two NumPy arrays.
Sample Solution:
Python Code:
import numpy as np
# Create two NumPy arrays
array1 = np.array([10, 20, 30, 40, 50])
array2 = np.array([100, 200, 300, 400, 500])
# Perform element-wise addition
result_array = array1 + array2
# Display the result
print(result_array)
Output:
[110 220 330 440 550]
Explanation:
In the exerciser above,
- Create two NumPy arrays, array1 and array2.
- The array1 + array2 expression performs element-wise addition.
- The result is stored in the result_array.
- The print(result_array) statement displays the resulting array.
We can apply the same approach for arrays of any shape and dimension. The + operator will perform element-wise addition on corresponding elements of the arrays.
Flowchart:
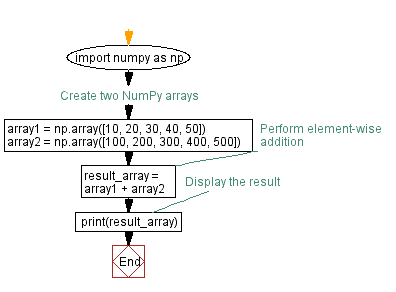
Python Code Editor:
Previous: Removing duplicate rows in Pandas DataFrame.
Next: Calculating the dot product of NumPy arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics