Generating a Pandas DataFrame from a NumPy array with custom column names in Python
Python Pandas Numpy: Exercise-2 with Solution
Create a DataFrame from a NumPy array with custom column names.
Sample Solution:
Python Code:
import pandas as pd
import numpy as np
# Create a NumPy array
numpy_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Define custom column names
column_names = ['Column1', 'Column2', 'Column3']
# Create a DataFrame with custom column names
df = pd.DataFrame(data=numpy_array, columns=column_names)
# Display the DataFrame
print(df)
Output:
Column1 Column2 Column3 0 1 2 3 1 4 5 6 2 7 8 9
Explanation:
- Importing Libraries:
import pandas as pd
import numpy as np
Imports the Pandas and NumPy libraries with the aliases "pd" and "np". - Creating a NumPy Array:
numpy_array = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) Creates a 2D NumPy array named "numpy_array". - Defining Custom Column Names:
column_names = ['Column1', 'Column2', 'Column3']
Defines custom column names in a list named "column_names". - Creating a DataFrame with Custom Column Names:
df = pd.DataFrame(data=numpy_array, columns=column_names)
Uses the pd.DataFrame constructor to create a DataFrame (df) from the NumPy array with specified column names. - Displaying the DataFrame:
print(df)
Flowchart:
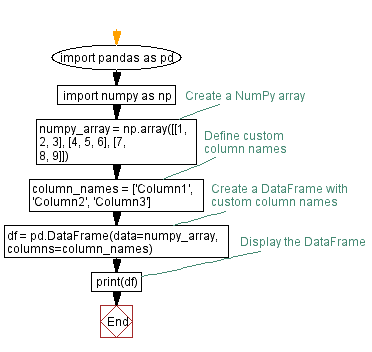
Python Code Editor:
Previous: Loading a CSV file into a Pandas DataFrame with Python.
Next: Selecting rows based on multiple conditions in Pandas DataFrame.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pandas_numpy/pandas_numpy-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics