Calculating the dot product of NumPy arrays
Calculate the dot product of two NumPy arrays.
Sample Solution-1:
Python Code:
import numpy as np
# Create two NumPy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Calculate the dot product
dot_product = np.dot(array1, array2)
# Display the result
print(dot_product)
Output:
32
Sample Solution-2:
Python Code:
import numpy as np
# Create two NumPy arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Calculate the dot product
dot_product = array1 @ array2
# Display the result
print(dot_product)
Output:
21
Flowchart:
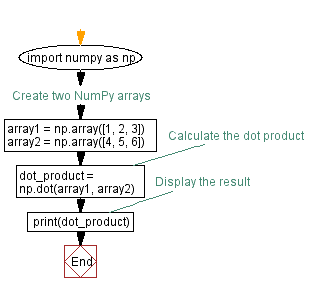
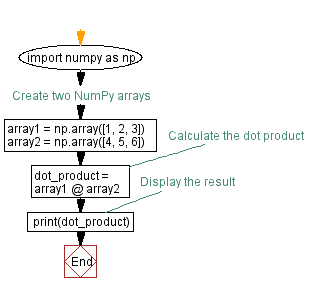
Python Code Editor:
Previous: Performing element-wise addition in NumPy arrays.
Next: Finding index of maximum and minimum values in a NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.