Finding index of maximum and minimum values in a NumPy array
Find the index of the maximum and minimum value in a NumPy array.
Sample Solution:
Python Code:
import numpy as np
# Create a NumPy array
array = np.array([10, 5, 8, 20, 3])
# Find the index of the maximum value
max_index = np.argmax(array)
# Find the index of the minimum value
min_index = np.argmin(array)
# Display the results
print("Index of maximum value:", max_index)
print("Index of minimum value:", min_index)
Output:
Index of maximum value: 3 Index of minimum value: 2
Explanation:
Here's a breakdown of the above code:
- First create a NumPy array “array”.
- The np.argmax(array) function returns the index of the maximum value in the array.
- The np.argmin(array) function returns the index of the minimum value in the array.
- The print() function displays the results.
Flowchart:
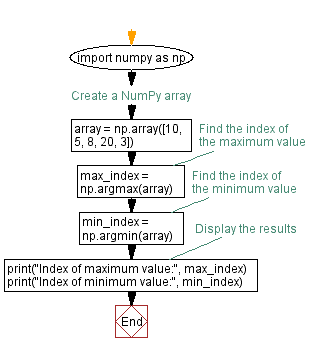
Python Code Editor:
Previous: Calculating the dot product of NumPy arrays.
Next: Reshaping a 1D NumPy array into a 2D array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics