Slicing and extracting a portion of a NumPy array
Python Pandas Numpy: Exercise-23 with Solution
Slice and extract a portion of a NumPy array.
Sample Solution:
Python Code:
import numpy as np
# Create a NumPy array
nums = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9])
# Slice and extract a portion of the array
portion = nums[2:6]
# Display the extracted portion
print(portion)
Output:
[3 4 5 6]
Explanation:
Here's a breakdown of the above code:
- We create a NumPy array "nums".
- The slice notation nums[2:6] is used to extract elements from index 2 to 5 (inclusive). Note that the ending index is exclusive in Python slicing.
- The extracted portion is stored in the variable "portion".
- The result is printed, showing the extracted portion of the array.
Flowchart:
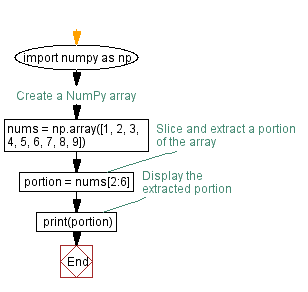
Python Code Editor:
Previous: Reshaping a 1D NumPy array into a 2D array.
Next: Concatenating NumPy Arrays Vertically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pandas_numpy/pandas_numpy-exercise-23.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics