Cumulative sum of a NumPy array
Python Pandas Numpy: Exercise-26 with Solution
Calculate the cumulative sum of a NumPy array.
Sample Solution:
Python Code:
import numpy as np
# Create a sample NumPy array
nums = np.array([1, 2, 3, -4, 5])
# Calculate the cumulative sum
cumulative_sum = np.cumsum(nums)
# Display the cumulative sum
print(cumulative_sum)
Output:
[1 3 6 2 7]
Explanation:
Here's a breakdown of the above code:
- First we create a sample NumPy array "nums".
- The np.cumsum(nums) function calculates the cumulative sum of the elements in the array.
- The resulting "cumulative_sum" is a NumPy array containing the cumulative sums.
- The result is printed, showing the cumulative sum of the array.
Flowchart:
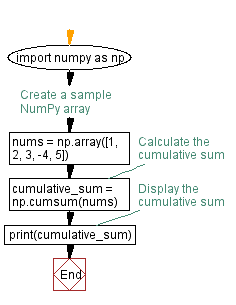
Python Code Editor:
Previous: Matrix Multiplication with NumPy.
Next: Generating and identifying unique values in a NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pandas_numpy/pandas_numpy-exercise-26.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics