Generating and identifying unique values in a NumPy array
Create a NumPy array with random values and find the unique values.
Sample Solution:
Python Code:
import numpy as np
# Create a NumPy array with random values
random_array = np.random.rand(10)
# Find unique values in the array
unique_values = np.unique(random_array)
# Display the original array and unique values
print("Original array:")
print(random_array)
print("\nUnique values:")
print(unique_values)
Output:
Original array: [0.20644509 0.07035208 0.12750982 0.19506758 0.21342963 0.33500047 0.58841512 0.52189153 0.97505776 0.02846753] Unique values: [0.02846753 0.07035208 0.12750982 0.19506758 0.20644509 0.21342963 0.33500047 0.52189153 0.58841512 0.97505776]
Explanation:
Here's a breakdown of the above code:
- np.random.rand(10) generates a 1D array with 10 random values between 0 and 1.
- np.unique(random_array) returns an array containing the unique values from random_array.
- The original array and unique values are printed to the console.
Flowchart:
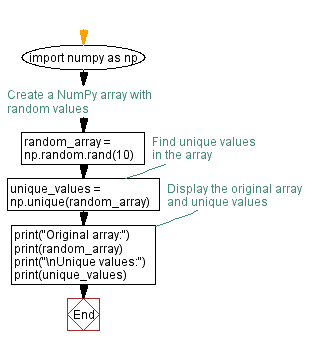
Python Code Editor:
Previous: Cumulative sum of a NumPy array.
Next: Sorting Pandas DataFrame by values: Python data manipulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.