Applying custom function to salary: Pandas DataFrame operation
Python Pandas Numpy: Exercise-29 with Solution
Apply a custom function to each element in a Pandas DataFrame.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['Imen', 'Karthika', 'Cosimo', 'Cathrine'],
'Age': [25, 30, 22, 35],
'Salary': [50000, 60000, 45000, 70000]}
df = pd.DataFrame(data)
# Define a custom function
def double_salary(salary):
return salary * 2
# Apply the custom function to the 'Salary' column
df['Double_Salary'] = df['Salary'].apply(double_salary)
# Display the DataFrame with the new column
print(df)
Output:
Name Age Salary Double_Salary 0 Imen 25 50000 100000 1 Karthika 30 60000 120000 2 Cosimo 22 45000 90000 3 Cathrine 35 70000 140000
Explanation:
Here's a breakdown of the above code:
- First we create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- Next we define a custom function "double_salary" that doubles the input value.
- The df['Salary'].apply(double_salary) line applies the custom function to each element in the 'Salary' column.
- The result is stored in a new column 'Double_Salary', which is added to the DataFrame.
- The DataFrame with the new column is printed.
Flowchart:
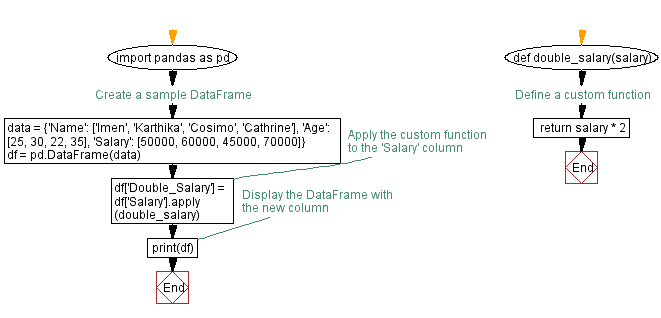
Python Code Editor:
Previous: Sorting Pandas DataFrame by values: Python data manipulation.
Next: Renaming columns in Pandas DataFrame.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pandas_numpy/pandas_numpy-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics