Renaming columns in Pandas DataFrame
Rename columns in a Pandas DataFrame.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['Imen', 'Karthika', 'Cosimo', 'Cathrine'],
'Age': [25, 30, 22, 35],
'Salary': [50000, 60000, 45000, 70000]}
df = pd.DataFrame(data)
# Rename columns
df.rename(columns={'Name': 'Full_Name', 'Age': 'Years', 'Salary': 'Income'}, inplace=True)
# Display the DataFrame with renamed columns
print(df)
Output:
Full_Name Years Income 0 Imen 25 50000 1 Karthika 30 60000 2 Cosimo 22 45000 3 Cathrine 35 70000
Explanation:
Here's a breakdown of the above code:
- First we create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- The df.rename(columns={'Name': 'Full_Name', 'Age': 'Years', 'Salary': 'Income'}, inplace=True) line renames the specified columns using a dictionary.
- The inplace=True argument modifies the DataFrame in place without the need to create a new DataFrame.
- The resulting DataFrame is displayed with renamed columns.
Flowchart:
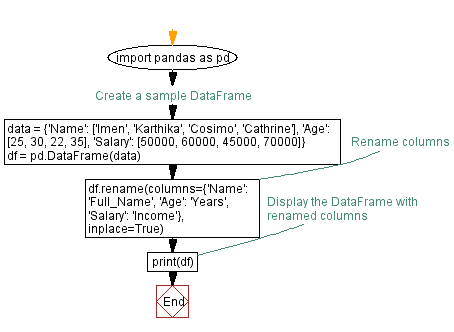
Python Code Editor:
Previous: Applying custom function to salary: Pandas DataFrame operation.
Next: Transposing DataFrame: Pandas data manipulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.