Transposing DataFrame: Pandas data manipulation
Create a new DataFrame by transposing an existing one.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame
data = {'Name': ['Imen', 'Karthika', 'Cosimo', 'Cathrine'],
'Age': [25, 30, 22, 35],
'Salary': [50000, 60000, 45000, 70000]}
df = pd.DataFrame(data)
# Transpose the DataFrame using transpose() method
transposed_df = df.transpose()
# Alternatively, you can use the .T attribute
# transposed_df = df.T
# Display the transposed DataFrame
print(transposed_df)
Output:
0 1 2 3 Name Imen Karthika Cosimo Cathrine Age 25 30 22 35 Salary 50000 60000 45000 70000
Explanation:
Here's a breakdown of the above code:
- First we create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- The df.transpose() method transposes the DataFrame, swapping rows and columns.
- Alternatively, you can use df.T for the same effect.
- The resulting transposed_df DataFrame is printed, showing the transposed layout.
Flowchart:
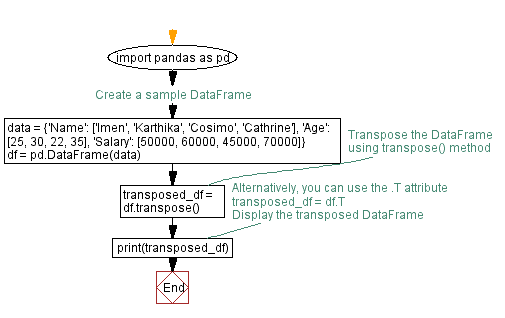
Python Code Editor:
Previous: Renaming columns in Pandas DataFrame.
Next: Merging Pandas DataFrames on multiple columns.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.