Extracting date and time from Pandas DateTime
Extract the date and time components from a DateTime column.
Sample Solution:
Python Code:
import pandas as pd
# Create a sample DataFrame with a DateTime column
data = {'DateTime': ['2012-01-01 08:30:00', '2012-01-02 12:45:00', '2012-01-03 18:15:00']}
df = pd.DataFrame(data)
# Convert the 'DateTime' column to a datetime type
df['DateTime'] = pd.to_datetime(df['DateTime'])
# Extract date and time components
df['Date'] = df['DateTime'].dt.date
df['Time'] = df['DateTime'].dt.time
# Display the DataFrame with extracted components
print(df)
Output:
DateTime Date Time 0 2012-01-01 08:30:00 2012-01-01 08:30:00 1 2012-01-02 12:45:00 2012-01-02 12:45:00 2 2012-01-03 18:15:00 2012-01-03 18:15:00
Explanation:
Here's a breakdown of the above code:
- First we create a sample DataFrame (df) with a 'DateTime' column containing strings representing date and time.
- Next we use pd.to_datetime() to convert the 'DateTime' column to a Pandas datetime type.
- The df['DateTime'].dt.date extracts the date component, and df['DateTime'].dt.time extracts the time component.
- New columns 'Date' and 'Time' are added to the DataFrame.
- The resulting DataFrame is printed.
Flowchart:
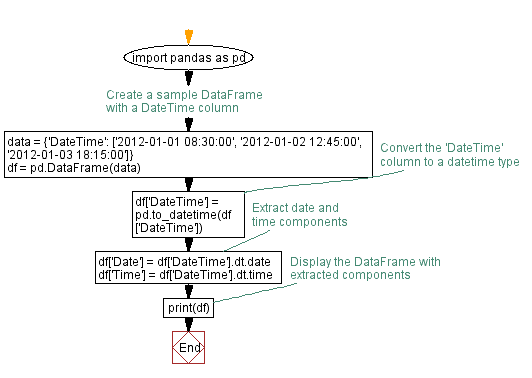
Python Code Editor:
Previous: Aggregating data in Pandas: Multiple functions example.
Next: Resampling Time-Series in a Pandas DataFrame.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.