Selecting the first and last 7 rows in a Pandas DataFrame
Select the first and last 7 rows of a Pandas DataFrame.
Sample Solution:
Python Code:
Output:
First 7 rows: Name Age Salary 0 Teodosija 26 50000 1 Sutton 32 60000 2 Taneli 25 45000 3 Ravshan 31 70000 4 Ross 28 55000 5 Alice 22 60000 6 Bob 35 70000 Last 7 rows: Name Age Salary 3 Ravshan 31 70000 4 Ross 28 55000 5 Alice 22 60000 6 Bob 35 70000 7 Charlie 30 55000 8 David 40 75000 9 Emily 28 65000
Explanation:
- Importing Pandas:
import pandas as pd
Imports the Pandas library and aliases it as "pd" for convenience. - Creating a Sample DataFrame:
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'Ravshan', 'Ross', 'Alice', 'Bob', 'Charlie', 'David', 'Emily'], 'Age': [26, 32, 25, 31, 28, 22, 35, 30, 40, 28], 'Salary': [50000, 60000, 45000, 70000, 55000, 60000, 70000, 55000, 75000, 65000]} df = pd.DataFrame(data)
Creates a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'. - Selecting the First 7 Rows:
selected_rows = pd.concat([df.head(7)])
Uses the head(7) method to select the first 7 rows of the DataFrame and stores it in selected_rows. - Displaying the First 7 Rows:
print("First 7 rows:") print(selected_rows)
Prints a message and displays the selected rows to the console. - Selecting the Last 7 Rows:
selected_rows = pd.concat([df.tail(7)])
Uses the tail(7) method to select the last 7 rows of the DataFrame and stores it in selected_rows. - Displaying the Last 7 Rows:
print("\nLast 7 rows:") print(selected_rows)
Prints a message and displays the selected rows to the console.
Flowchart:
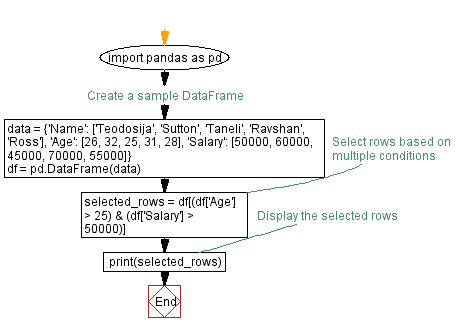
Python Code Editor:
Previous: Selecting rows based on multiple conditions in Pandas DataFrame.
Next: Filtering rows based on a column condition in Pandas DataFrame.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.