Creating a new column with NumPy operation in Pandas DataFrame
Create a new column in a Pandas DataFrame based on the result of a NumPy operation.
Sample Solution:
Python Code:
import pandas as pd
import numpy as np
# Create a sample DataFrame
data = {'Name': ['Teodosija', 'Sutton', 'Taneli', 'Ravshan', 'Ross'],
'Age': [26, 32, 25, 31, 28],
'Salary': [50000, 60000, 45000, 70000, 55000]}
df = pd.DataFrame(data)
# Perform a NumPy operation (e.g., multiply Age by 2) and create a new column
df['Double_Age'] = np.multiply(df['Age'], 2)
print("New Dataframe:")
# Display the DataFrame with the new column
print(df)
Output:
New Dataframe: Name Age Salary Double_Age 0 Teodosija 26 50000 52 1 Sutton 32 60000 64 2 Taneli 25 45000 50 3 Ravshan 31 70000 62 4 Ross 28 55000 56
Explanation:
In the exerciser above -
- First create a sample DataFrame (df) with columns 'Name', 'Age', and 'Salary'.
- The NumPy operation np.multiply(df['Age'], 2) multiplies each element in the 'Age' column by 2.
- Create a new column 'Double_Age' in the DataFrame and assign the result of the NumPy operation to it.
- The DataFrame is then printed to the console, showing the newly added 'Double_Age' column.
Flowchart:
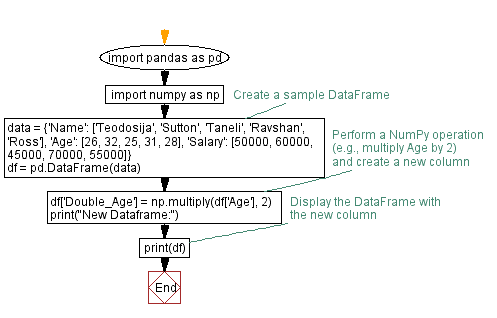
Python Code Editor:
Previous: Selecting the first and last 7 rows in a Pandas DataFrame.
Next: Merging DataFrames based on a common column in Pandas.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.