Python: Find the strings in a list, starting with a given prefix
Find Strings with Prefix
Write a Python program to find strings in a given list starting with a given prefix.
Input: [( ca,('cat', 'car', 'fear', 'center'))] Output: ['cat', 'car'] Input: [(do,('cat', 'dog', 'shatter', 'donut', 'at', 'todo'))] Output: ['dog', 'donut']
Visual Presentation:
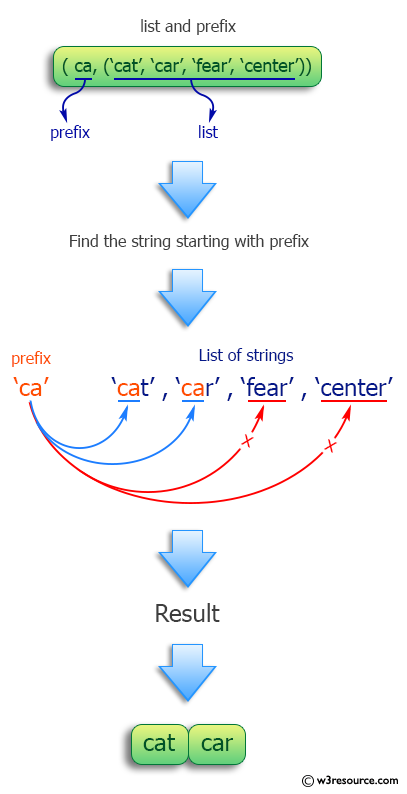
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' and a prefix 'prefix' as input
def test(strs, prefix):
# Use a list comprehension to filter strings in 'strs' that start with the given 'prefix'
return [s for s in strs if s.startswith(prefix)]
# Create a list of strings 'strs' with specific elements
strs = ['cat', 'car', 'fear', 'center']
# Assign a specific prefix 'prefix' to the variable
prefix = "ca"
# Print the original list of strings
print("Original strings:")
print(strs)
# Print the starting prefix
print("Starting prefix:", prefix)
# Print a message indicating the operation to be performed on the list
print("Strings in the said list starting with a given prefix:")
# Print the result of the test function applied to the 'strs' list with the given prefix
print(test(strs, prefix))
# Create a different list of strings 'strs' with specific elements
strs = ['cat', 'dog', 'shatter', 'donut', 'at', 'todo']
# Assign a different prefix 'prefix' to the variable
prefix = "do"
# Print the original list of strings
print("\nOriginal strings:")
print(strs)
# Print the updated starting prefix
print("Starting prefix:", prefix)
# Print a message indicating the operation to be performed on the list
print("Strings in the said list starting with a given prefix:")
# Print the result of the test function applied to the modified 'strs' list with the updated prefix
print(test(strs, prefix))
Sample Output:
Original strings: ['cat', 'car', 'fear', 'center'] Starting prefix: ca Strings in the said list starting with a given prefix: ['cat', 'car'] Original strings: ['cat', 'dog', 'shatter', 'donut', 'at', 'todo'] Starting prefix: do Strings in the said list starting with a given prefix: ['dog', 'donut']
Flowchart:
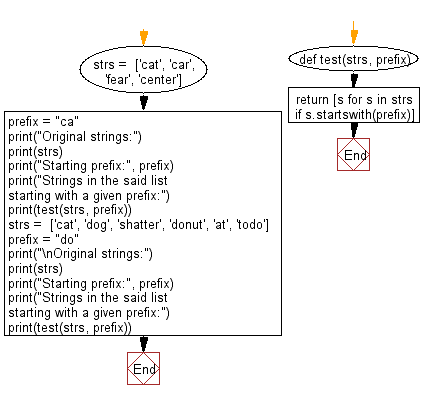
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Test whether the given strings are palindromes.
Next: Find the lengths of a list of non-empty strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics