Python: Find the lengths of a list of non-empty strings
Python Programming Puzzles: Exercise-14 with Solution
Write a Python program to find the length of a given list of non-empty strings.
Input: ['cat', 'car', 'fear', 'center'] Output: [3, 3, 4, 6] Input: ['cat', 'dog', 'shatter', 'donut', 'at', 'todo', ''] Output: [3, 3, 7, 5, 2, 4, 0]
Visual Presentation:
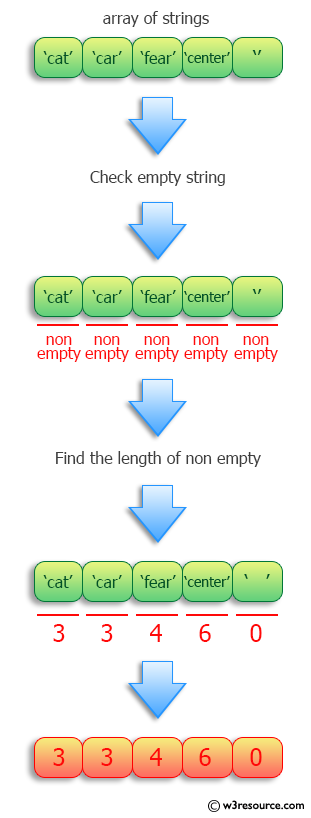
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Use the map function to apply the len function to each string in 'strs', and convert the result to a list
return [*map(len, strs)]
# Create a list of strings 'strs' with specific elements
strs = ['cat', 'car', 'fear', 'center']
# Print the original list of strings
print("Original strings:")
print(strs)
# Print a message indicating the operation to be performed on the list
print("Lengths of the said list of non-empty strings:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
# Create a different list of strings 'strs' with specific elements
strs = ['cat', 'dog', 'shatter', 'donut', 'at', 'todo', '']
# Print the original list of strings
print("\nOriginal strings:")
print(strs)
# Print a message indicating the operation to be performed on the list
print("Lengths of the said list of non-empty strings:")
# Print the result of the test function applied to the modified 'strs' list
print(test(strs))
Sample Output:
Original strings: ['cat', 'car', 'fear', 'center'] Lengths of the said list of non-empty strings: [3, 3, 4, 6] Original strings: ['cat', 'dog', 'shatter', 'donut', 'at', 'todo', ''] Lengths of the said list of non-empty strings: [3, 3, 7, 5, 2, 4, 0]
Flowchart:
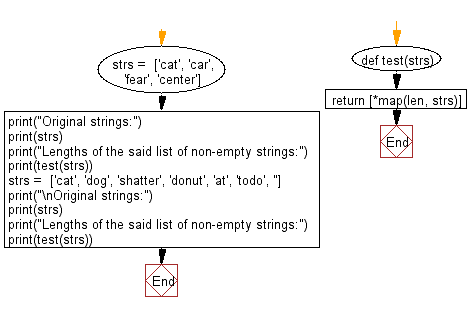
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the strings in a list, starting with a given prefix.
Next: Find the longest string of a list of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics