Python: Determine the direction ('increasing' or 'decreasing') of monotonic sequence numbers
Python Programming Puzzles: Exercise-20 with Solution
Monotonic sequences are sequences, which constantly increase or constantly decrease.
Write a Python program to determine the direction ('increasing' or 'decreasing') of monotonic sequence numbers.
Input: [1, 2, 3, 4, 5, 6] Output: Increasing. Input: [6, 5, 4, 3, 2, 1] Output: Decreasing. Input: [19, 19, 5, 5, 5, 5, 5] Output: Not a monotonic sequence!
Visual Presentation:
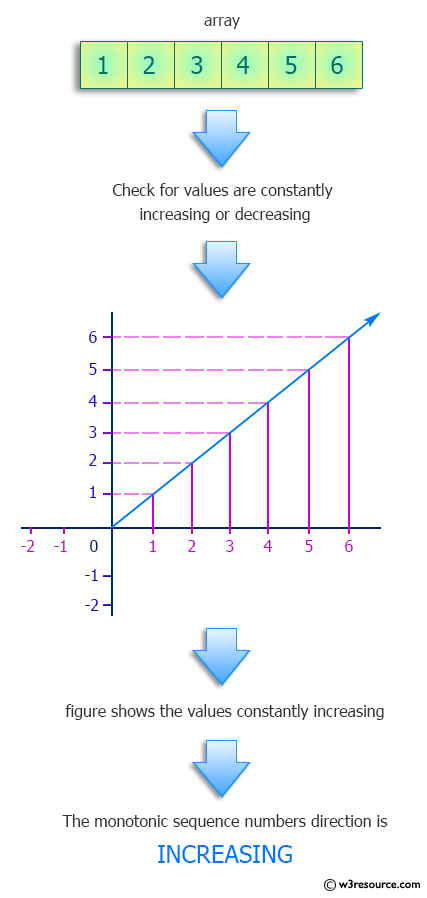
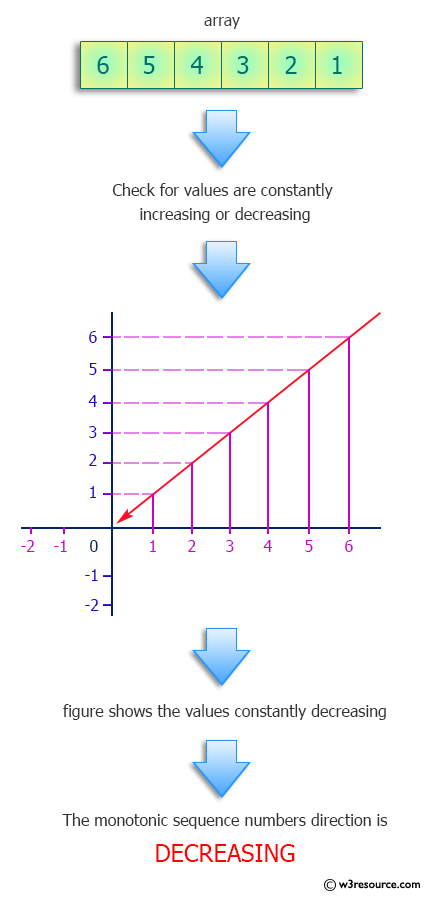
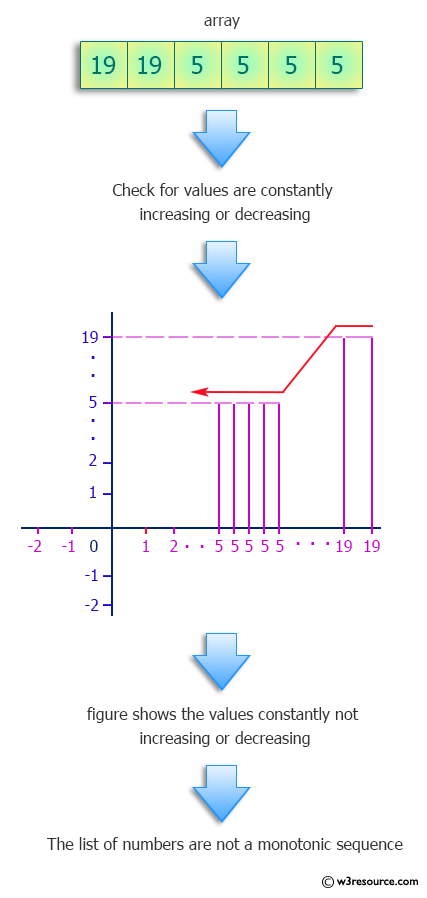
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Check if all elements in the list 'nums' are in increasing order
if all(nums[i] < nums[i + 1] for i in range(len(nums) - 1)):
return "Increasing."
# Check if all elements in the list 'nums' are in decreasing order
elif all(nums[i + 1] < nums[i] for i in range(len(nums) - 1)):
return "Decreasing."
else:
return "Not a monotonic sequence!"
# Assign a specific list of numbers 'nums' to the variable
nums = [1, 2, 3, 4, 5, 6]
# Print the original list of numbers 'nums'
print("Original list:")
print(nums)
# Print a message indicating the operation to be performed
print("Check the direction ('increasing' or 'decreasing') of the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign a different list of numbers 'nums' to the variable
nums = [6, 5, 4, 3, 2, 1]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("Check the direction ('increasing' or 'decreasing') of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
# Assign another different list of numbers 'nums' to the variable
nums = [19, 19, 5, 5, 5, 5, 5]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("Check the direction ('increasing' or 'decreasing') of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
Sample Output:
Original list: [1, 2, 3, 4, 5, 6] Check the direction ('increasing' or 'decreasing') of the said list: Increasing. Original list: [6, 5, 4, 3, 2, 1] Check the direction ('increasing' or 'decreasing') of the said list: Decreasing. Original list: [19, 19, 5, 5, 5, 5, 5] Check the direction ('increasing' or 'decreasing') of the said list: Not a monotonic sequence!
Flowchart:
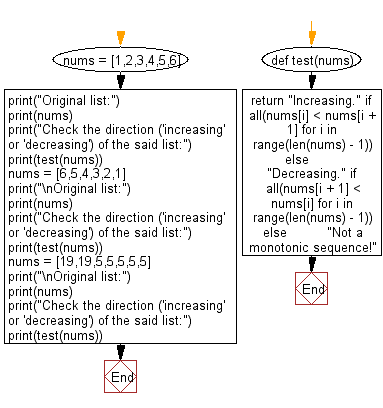
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Split a string into strings if there is a space in the string, otherwise split on commas, otherwise the list of lowercase letters with odd order.
Next: Determine, for each string in a list, whether the last character is an isolated letter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-20.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics