Python: Create a list whose ith element is the maximum of the first i elements of the input list
Max of First i Elements
Write a Python program to create a list whose ith element is the maximum of the first i elements from an input list.
Input: [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0] Output: [0, 0, 3, 8, 8, 9, 9, 14, 14, 14, 14, 14, 14, 17, 41, 41, 41, 41, 41, 41] Input: [6, 5, 4, 3, 2, 1] Output: [6, 6, 6, 6, 6, 6] Input: [1, 19, 5, 15, 5, 25, 5] Output: [1, 19, 19, 19, 19, 25, 25]
Visual Presentation:
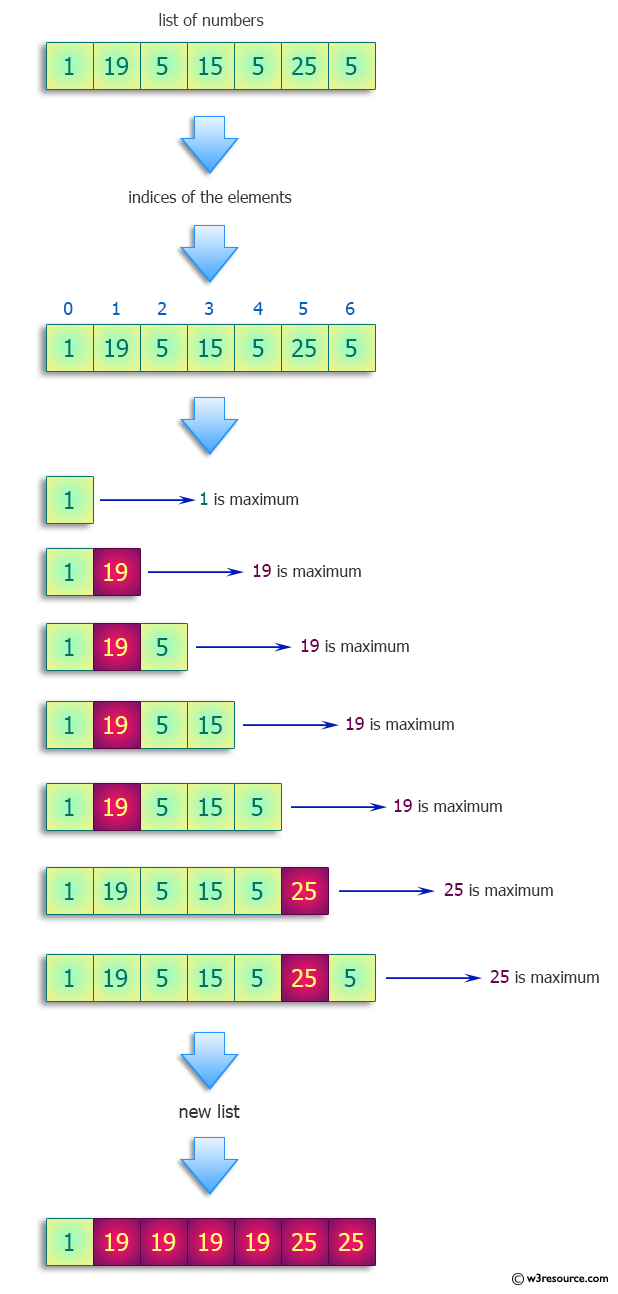
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# List comprehension to create a list of maximum values for each prefix of the input list
# Iterate through the indices from 1 to the length of 'nums' + 1
# For each index 'i', find the maximum value in the prefix nums[:i]
return [max(nums[:i]) for i in range(1, len(nums) + 1)]
# Assign a specific list of numbers 'nums' to the variable
nums = [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0]
# Print the original list of numbers 'nums'
print("Original list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign a different list of numbers 'nums' to the variable
nums = [6, 5, 4, 3, 2, 1]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
# Assign another list of numbers 'nums' to the variable
nums = [1, 19, 5, 15, 5, 25, 5]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
Sample Output:
Original list: [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0] List whose ith element is the maximum of the first i elements of the said list: [0, 0, 3, 8, 8, 9, 9, 14, 14, 14, 14, 14, 14, 17, 41, 41, 41, 41, 41, 41] Original list: [6, 5, 4, 3, 2, 1] List whose ith element is the maximum of the first i elements of the said list: [6, 6, 6, 6, 6, 6] Original list: [1, 19, 5, 15, 5, 25, 5] List whose ith element is the maximum of the first i elements of the said list: [1, 19, 19, 19, 19, 25, 25]
Flowchart:
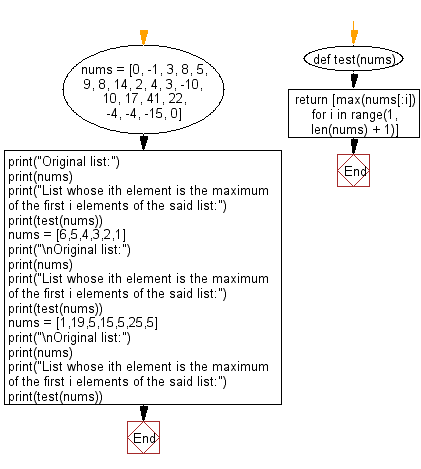
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# List comprehension to create a list of maximum values for each prefix of the input list
# Iterate through the indices from 0 to the length of 'nums'
# For each index 'i', find the maximum value in the prefix nums[:i+1]
return [max(nums[:i+1]) for i in range(len(nums))]
# Assign a specific list of numbers 'nums' to the variable
nums = [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0]
# Print the original list of numbers 'nums'
print("Original list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign a different list of numbers 'nums' to the variable
nums = [6, 5, 4, 3, 2, 1]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
# Assign another list of numbers 'nums' to the variable
nums = [1, 19, 5, 15, 5, 25, 5]
# Print the original list of numbers 'nums'
print("\nOriginal list:")
print(nums)
# Print a message indicating the operation to be performed
print("List whose ith element is the maximum of the first i elements of the said list:")
# Print the result of the test function applied to the updated 'nums' list
print(test(nums))
Sample Output:
Original list: [0, -1, 3, 8, 5, 9, 8, 14, 2, 4, 3, -10, 10, 17, 41, 22, -4, -4, -15, 0] List whose ith element is the maximum of the first i elements of the said list: [0, 0, 3, 8, 8, 9, 9, 14, 14, 14, 14, 14, 14, 17, 41, 41, 41, 41, 41, 41] Original list: [6, 5, 4, 3, 2, 1] List whose ith element is the maximum of the first i elements of the said list: [6, 6, 6, 6, 6, 6] Original list: [1, 19, 5, 15, 5, 25, 5] List whose ith element is the maximum of the first i elements of the said list: [1, 19, 19, 19, 19, 25, 25]
Flowchart:
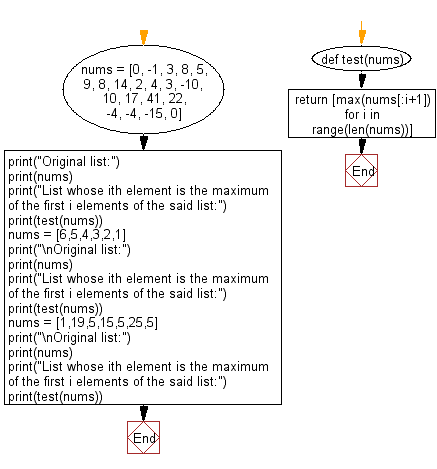
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the indices for which the numbers in the list drops.
Next: Find the XOR of two given strings interpreted as binary numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics