Python: Select a string from a given list of strings with the most unique characters
Python Programming Puzzles: Exercise-28 with Solution
Write a Python program to select a string from a given list of strings with the most unique characters.
Input: ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique'] Output: abcdefhijklmnop Input: ['Green', 'Red', 'Orange', 'Yellow', '', 'White'] Output: Orange
Visual Presentation:
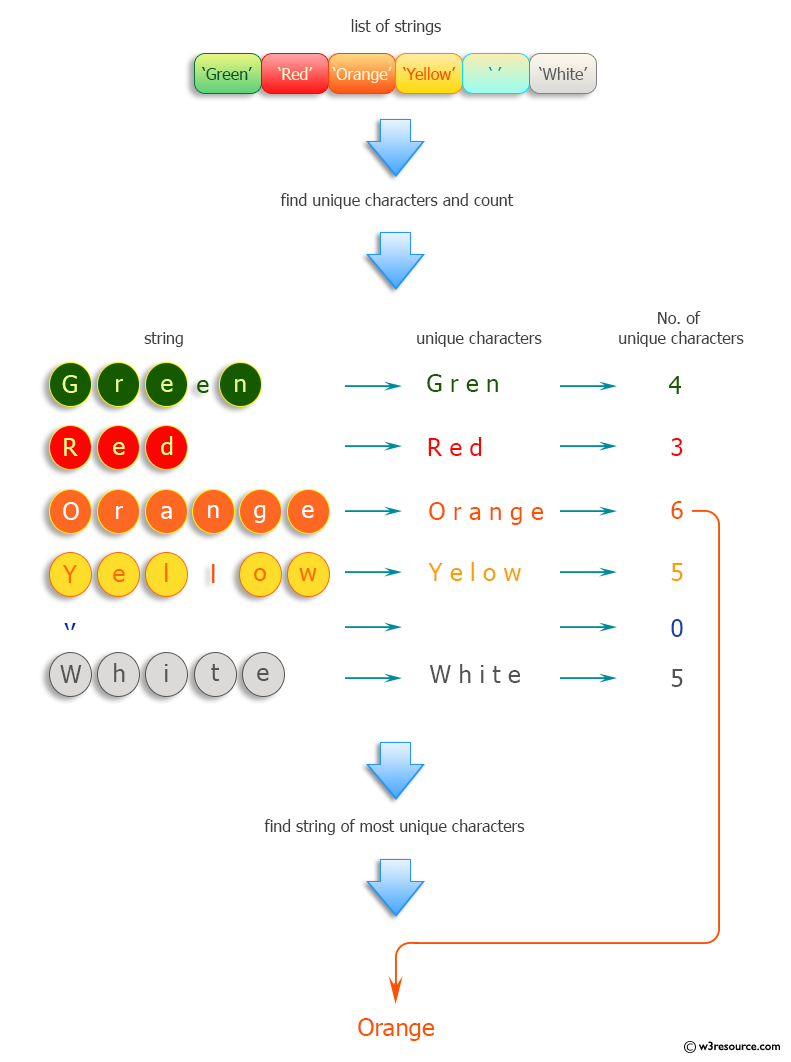
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Use the max function to find the string with the most unique characters
# The key argument specifies a lambda function that calculates the length of the set of characters in each string
return max(strs, key=lambda x: len(set(x)))
# Assign a specific list of strings 'strs' to the variable
strs = ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique']
# Print the original list of strings 'strs'
print("Original list:")
print(strs)
# Print a message indicating the operation to be performed
print("Select a string from the said list of strings with the most unique characters:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
# Assign another specific list of strings 'strs' to the variable
strs = ['Green', 'Red', 'Orange', 'Yellow', '', 'White']
# Print the original list of strings 'strs'
print("\nOriginal list:")
print(strs)
# Print a message indicating the operation to be performed
print("Select a string from the said list of strings with the most unique characters:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
Sample Output:
Original list: ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique'] Select a string from the said list of strings with the most unique characters: abcdefhijklmnop Original list: ['Green', 'Red', 'Orange', 'Yellow', '', 'White'] Select a string from the said list of strings with the most unique characters: Orange
Flowchart:
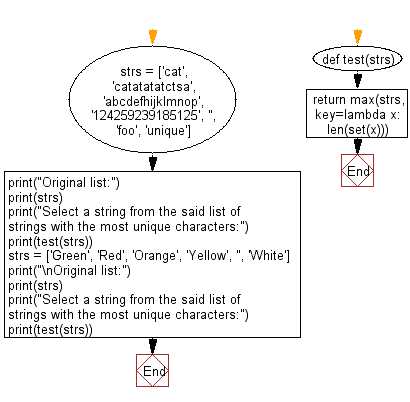
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Initialize variables to track the largest set, its length, and the corresponding string
largest_set = {}
n = 0
largest = None
# Iterate over each phrase in the list of strings 'strs'
for phrase in strs:
# Calculate the difference in lengths between the current largest set and the set of the current phrase
diff = len(largest_set) - len(set(phrase))
# Compare the differences and update variables accordingly
if diff < 0:
largest_set = set(phrase)
largest = phrase
n = len(largest_set)
elif diff == 0:
if n < len(set(phrase)):
largest_set = set(phrase)
largest = phrase
n = len(largest_set)
else:
pass
# Return the string with the most unique characters
return largest
# Assign a specific list of strings 'strs' to the variable
strs = ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique']
# Print the original list of strings 'strs'
print("Original list:")
print(strs)
# Print a message indicating the operation to be performed
print("Select a string from the said list of strings with the most unique characters:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
# Assign another specific list of strings 'strs' to the variable
strs = ['Green', 'Red', 'Orange', 'Yellow', '', 'White']
# Print the original list of strings 'strs'
print("\nOriginal list:")
print(strs)
# Print a message indicating the operation to be performed
print("Select a string from the said list of strings with the most unique characters:")
# Print the result of the test function applied to the 'strs' list
print(test(strs))
Sample Output:
Original list: ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique'] Select a string from the said list of strings with the most unique characters: abcdefhijklmnop Original list: ['Green', 'Red', 'Orange', 'Yellow', '', 'White'] Select a string from the said list of strings with the most unique characters: Orange
Flowchart:
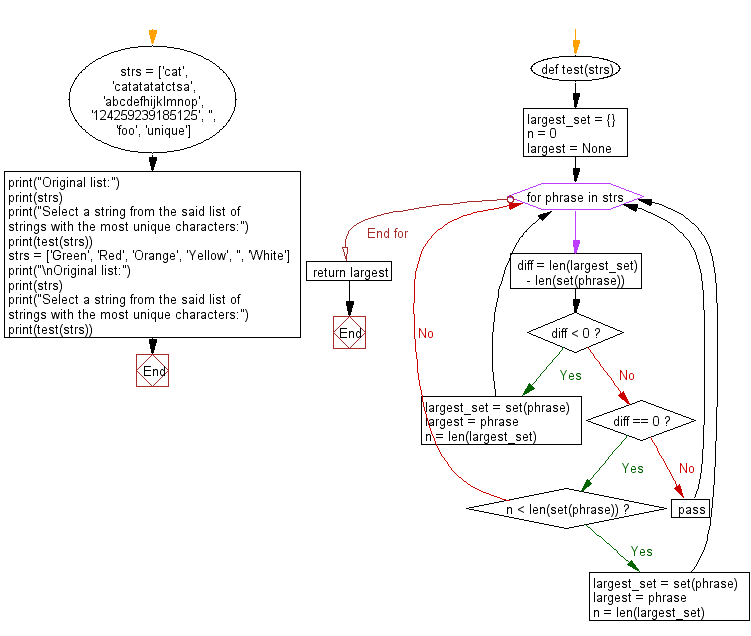
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find x that minimizes mean squared deviation from a given list of numbers.
Next: Find the indices of two numbers that sum to 0 in a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-28.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics