Python: Find the sum of the numbers among the first k with more than 2 digits
Python Programming Puzzles: Exercise-34 with Solution
Write a Python program to find the sum of the numbers in a given list among the first k with more than 2 digits.
Input: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 4 Output: 0 Input: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 6 Output: 108 Input: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 5 Output: 331 Input: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 1 Output: 114
Visual Presentation:
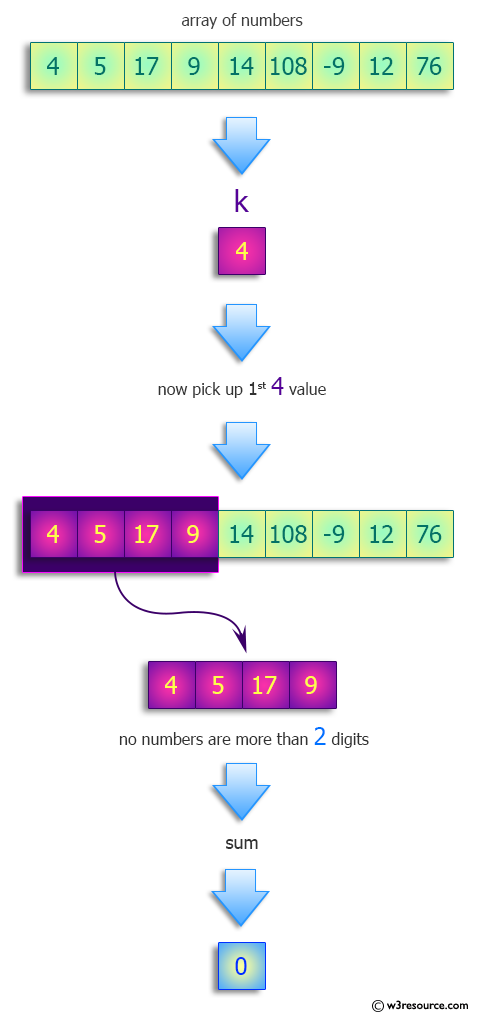
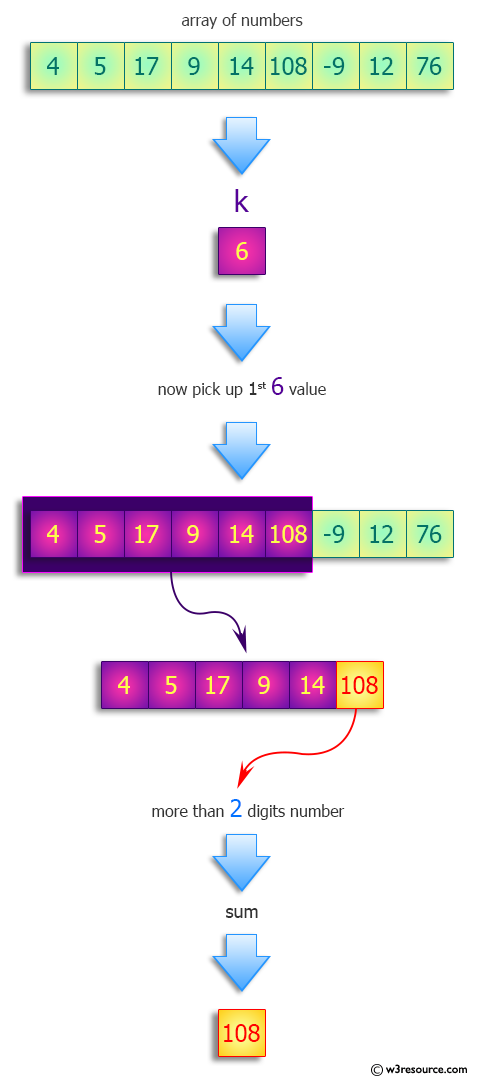
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a list of numbers 'nums' and an integer 'k' as input
def test(nums, k):
# Initialize a variable 's' to store the sum of numbers meeting the specified conditions
s = 0
# Iterate through the first 'k' elements in 'nums'
for i in range(len(nums))[:k]:
# Check if the absolute value of the current number has more than 2 digits
if len(str(abs(nums[i]))) > 2:
# Add the current number to the sum 's'
s = s + nums[i]
# Return the final sum 's'
return s
# Assign a specific list of numbers 'nums' to the variable
nums = [4, 5, 17, 9, 14, 108, -9, 12, 76]
# Print a message indicating the operation to be performed
print("Original list:", nums)
# Assign a specific value 'K' to the variable
K = 4
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Assign another specific value 'K' to the variable
K = 6
# Print a message indicating the value of 'K'
print("\nOriginal list:", nums)
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Assign another specific list of numbers 'nums' to the variable
nums = [114, 215, -117, 119, 14, 108, -9, 12, 76]
# Print a message indicating the operation to be performed
print("\nOriginal list:", nums)
# Assign another specific value 'K' to the variable
K = 5
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Print an additional message indicating the original list
print("\nOriginal list:", nums)
# Assign another specific value 'K' to the variable
K = 1
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
Sample Output:
Original list: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 4 sum of the numbers among the first k with more than 2 digits 0 Original list: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 6 sum of the numbers among the first k with more than 2 digits 108 Original list: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 5 sum of the numbers among the first k with more than 2 digits 331 Original list: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 1 sum of the numbers among the first k with more than 2 digits 114
Flowchart:
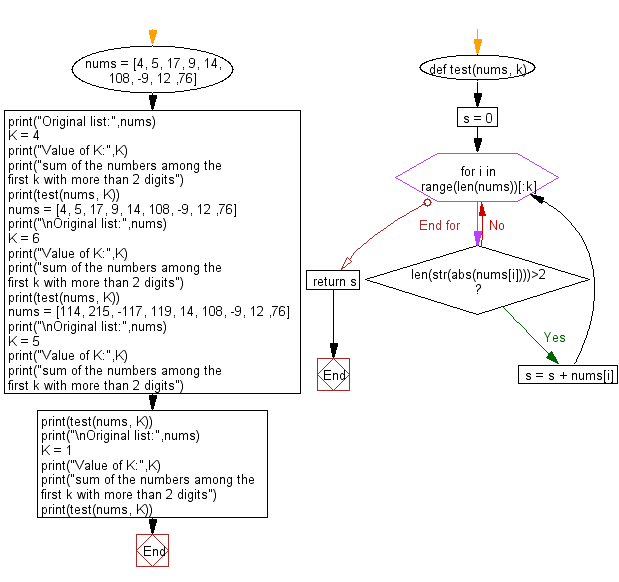
Sample Solution-2:
Python Code:
#License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' and an integer 'k' as input
def test(nums, k):
# Use a generator expression to sum numbers meeting the specified conditions in the first 'k' elements of 'nums'
return sum(n for n in nums[:k] if len(str(abs(n))) > 2)
# Assign a specific list of numbers 'nums' to the variable
nums = [4, 5, 17, 9, 14, 108, -9, 12, 76]
# Print a message indicating the operation to be performed
print("Original list:", nums)
# Assign a specific value 'K' to the variable
K = 4
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Assign another specific value 'K' to the variable
K = 6
# Print a message indicating the value of 'K'
print("\nOriginal list:", nums)
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Assign another specific list of numbers 'nums' to the variable
nums = [114, 215, -117, 119, 14, 108, -9, 12, 76]
# Print a message indicating the operation to be performed
print("\nOriginal list:", nums)
# Assign another specific value 'K' to the variable
K = 5
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
# Print an additional message indicating the original list
print("\nOriginal list:", nums)
# Assign another specific value 'K' to the variable
K = 1
# Print a message indicating the value of 'K'
print("Value of K:", K)
# Print a message indicating the operation to be performed
print("Sum of the numbers among the first k with more than 2 digits:")
# Print the result of the test function applied to 'nums' and 'K'
print(test(nums, K))
Sample Output:
Original list: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 4 sum of the numbers among the first k with more than 2 digits 0 Original list: [4, 5, 17, 9, 14, 108, -9, 12, 76] Value of K: 6 sum of the numbers among the first k with more than 2 digits 108 Original list: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 5 sum of the numbers among the first k with more than 2 digits 331 Original list: [114, 215, -117, 119, 14, 108, -9, 12, 76] Value of K: 1 sum of the numbers among the first k with more than 2 digits 114
Flowchart:
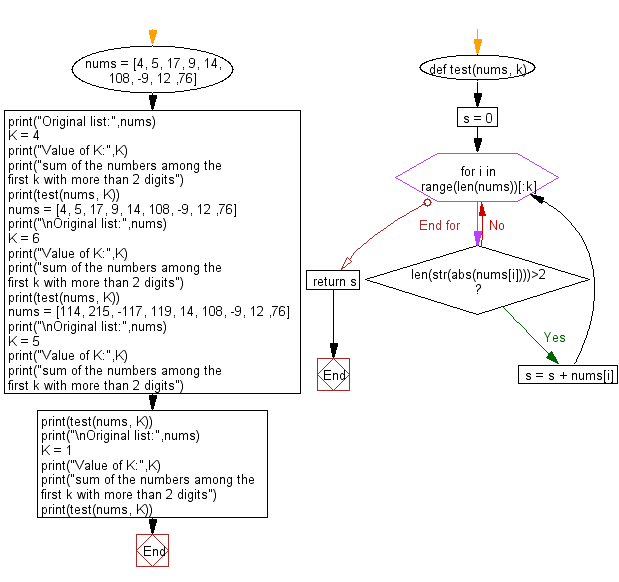
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the positions of all uppercase vowels (not counting Y) in even indices.
Next: Product of the odd digits in n, or 0 if there aren't any.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-34.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics