Python: Find the number of stones in each pile
Python Programming Puzzles: Exercise-4 with Solution
We are making n stone piles! The first pile has n stones. If n is even, then all piles have an even number of stones. If n is odd, all piles have an odd number of stones. Each pile must more stones than the previous pile but as few as possible. Write a Python program to find the number of stones in each pile.
Input: 2 Output: [2, 4] Input: 10 Output: [10, 12, 14, 16, 18, 20, 22, 24, 26, 28] Input: 3 Output: [3, 5, 7] Input: 17 Output: [17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39, 41, 43, 45, 47, 49]
Visual Presentation:
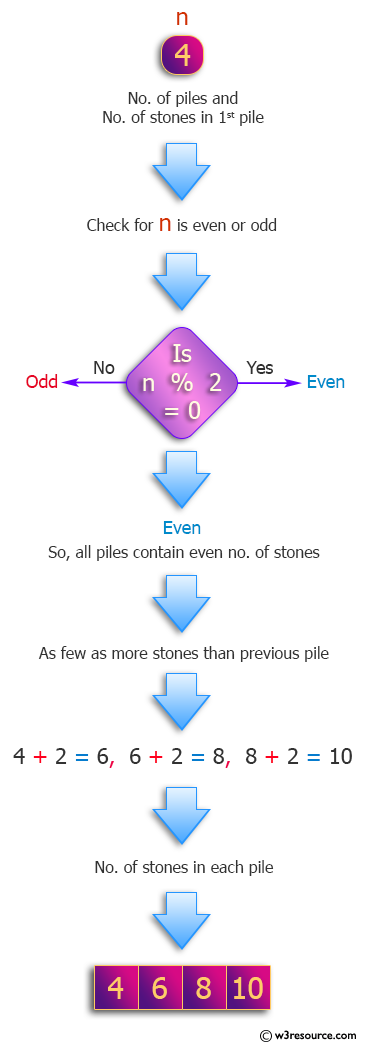
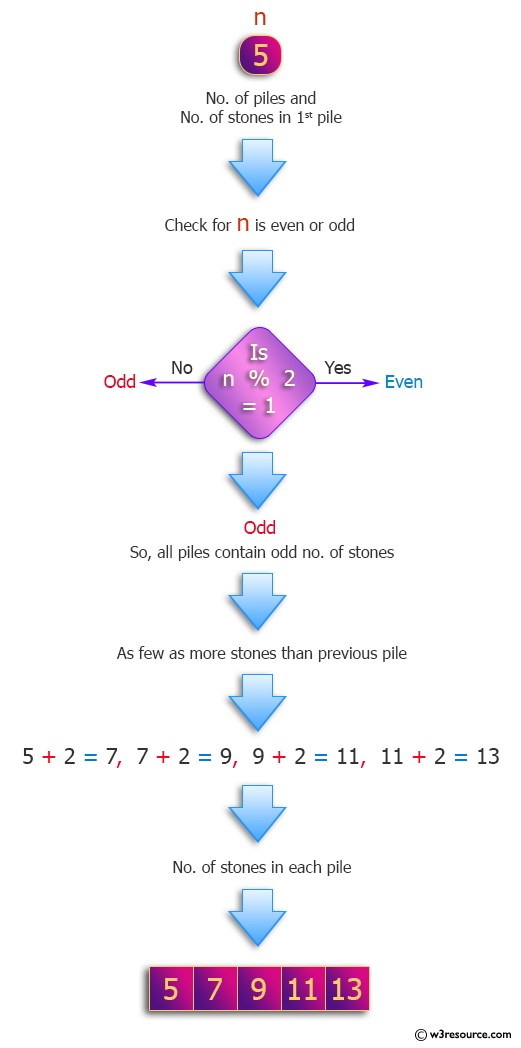
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes an integer 'n' as input
def test(n):
# Use a list comprehension to generate a list of values: n + 2 * i for i in the range from 0 to n-1
return [n + 2 * i for i in range(n)]
# Assign a specific integer 'n' to the variable
n = 2
# Print the number of piles
print("Number of piles:", n)
# Print the header for the output
print("Number of stones in each pile:")
# Print the result of the test function applied to the integer 'n'
print(test(n))
# Assign a different integer 'n' to the variable
n = 10
# Print the number of piles
print("\nNumber of piles:", n)
# Print the header for the output
print("Number of stones in each pile:")
# Print the result of the test function applied to the modified integer 'n'
print(test(n))
# Assign another integer 'n' to the variable
n = 3
# Print the number of piles
print("\nNumber of piles:", n)
# Print the header for the output
print("Number of stones in each pile:")
# Print the result of the test function applied to the modified integer 'n'
print(test(n))
# Assign yet another integer 'n' to the variable
n = 17
# Print the number of piles
print("\nNumber of piles:", n)
# Print the header for the output
print("Number of stones in each pile:")
# Print the result of the test function applied to the modified integer 'n'
print(test(n))
Sample Output:
Number of piles: 2 Number of stones in each pile: [2, 4] Number of piles: 10 Number of stones in each pile: [10, 12, 14, 16, 18, 20, 22, 24, 26, 28] Number of piles: 3 Number of stones in each pile: [3, 5, 7] Number of piles: 17 Number of stones in each pile: [17, 19, 21, 23, 25, 27, 29, 31, 33, 35, 37, 39
Flowchart:
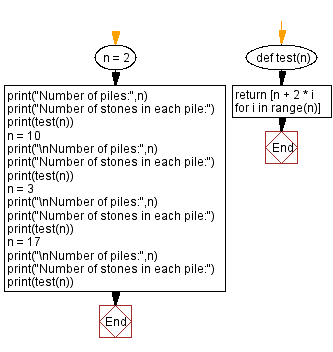
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Whether an integer greater than 4^4 which is 4 mod 34.
Next: Check the nth-1 string is a proper substring of nth string of a given list of strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics