Python: Find the set of distinct characters in a string, ignoring case
Distinct Characters in String
Write a Python program to find the set of distinct characters in a given string, ignoring case.
Input: HELLO Output: ['h', 'o', 'l', 'e'] Input: HelLo Output: ['h', 'o', 'l', 'e'] Input: Ignoring case Output: ['s', 'n', 'c', 'o', 'e', 'i', 'r', 'g', 'a', ' ']
Visual Presentation:
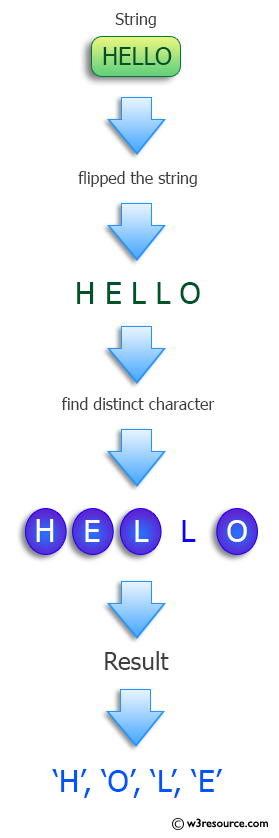
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Convert the input string to lowercase, create a set of distinct characters, and return it as a list
return [*set(strs.lower())]
# Assign a specific string 'strs' to the variable
strs = "HELLO"
# Print a message indicating the original string
print("Original string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "HelLo"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "Ignoring case"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
Sample Output:
Original string: HELLO Set of distinct characters in the said string, ignoring case: ['o', 'e', 'l', 'h'] Original string: HelLo Set of distinct characters in the said string, ignoring case: ['o', 'e', 'l', 'h'] Original string: Ignoring case Set of distinct characters in the said string, ignoring case: ['o', ' ', 'i', 'r', 'e', 'g', 'a', 'n', 'c', 's']
Flowchart:
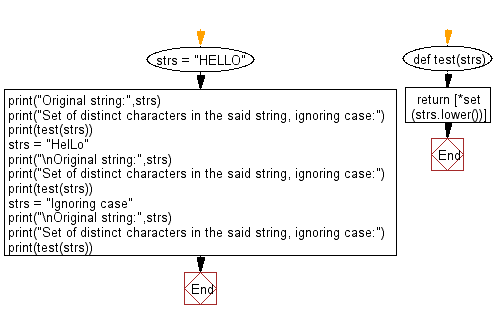
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Convert the input string to lowercase, create a set of distinct characters, and convert it back to a list
return list(set(strs.lower()))
# Assign a specific string 'strs' to the variable
strs = "HELLO"
# Print a message indicating the original string
print("Original string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "HelLo"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "Ignoring case"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Set of distinct characters in the said string, ignoring case:")
# Print the result of the test function applied to 'strs'
print(test(strs))
Sample Output:
Original string: HELLO Set of distinct characters in the said string, ignoring case: ['h', 'o', 'l', 'e'] Original string: HelLo Set of distinct characters in the said string, ignoring case: ['h', 'o', 'l', 'e'] Original string: Ignoring case Set of distinct characters in the said string, ignoring case: ['s', 'n', 'c', 'o', 'e', 'i', 'r', 'g', 'a', ' ']
Flowchart:
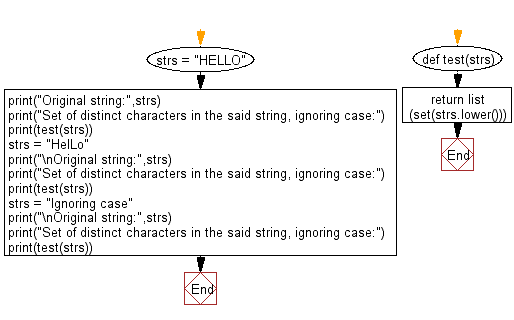
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sort numbers based on strings.
Next: Find all words in a given string with n consonants.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics