Python: Find the minimum even value and its index from a given array of numbers
Python Programming Puzzles: Exercise-46 with Solution
Given an array of numbers representing a branch on a binary tree, write a Python program to find the minimum even value and its index. In the case of a tie, return the smallest index. If there are no even numbers, the answer is [].
Input: [1, 9, 4, 6, 10, 11, 14, 8] Output: Minimum even value and its index of the said array of numbers: [4, 2] Input: [1, 7, 4, 4, 9, 2] Output: Minimum even value and its index of the said array of numbers: [2, 5] Input: [1, 7, 7, 5, 9] Output: Minimum even value and its index of the said array of numbers: []
Visual Presentation:
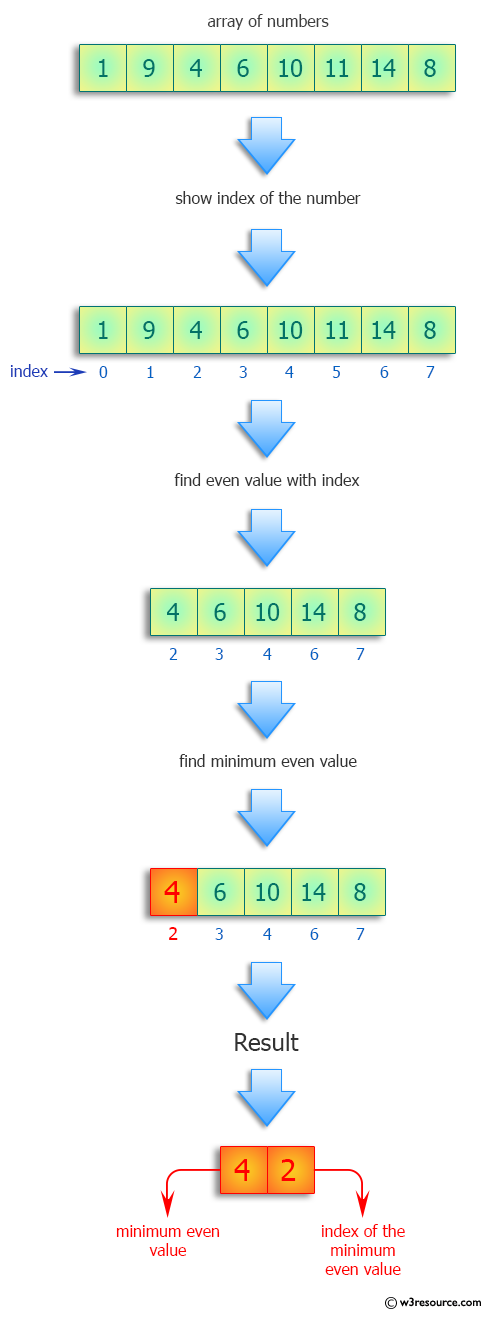
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Check if any element in 'nums' is even
if any(n % 2 == 0 for n in nums):
# If there is an even number, find the minimum even value and its index
return min([v, i] for i, v in enumerate(nums) if v % 2 == 0)
else:
# If there are no even numbers, return an empty list
return []
# Assign a specific list of numbers to the variable 'nums'
nums = [1, 9, 4, 6, 10, 11, 14, 8]
# Print a message indicating the original list of numbers
print("Original list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the minimum even value and its index
print("Minimum even value and its index of the said array of numbers:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1, 7, 4, 4, 9, 2]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the minimum even value and its index
print("Minimum even value and its index of the said array of numbers:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1, 7, 7, 5, 9]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the minimum even value and its index
print("Minimum even value and its index of the said array of numbers:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list: [1, 9, 4, 6, 10, 11, 14, 8] Minimum even value and its index of the said array of numbers: [4, 2] Original list: [1, 7, 4, 4, 9, 2] Minimum even value and its index of the said array of numbers: [2, 5] Original list: [1, 7, 7, 5, 9] Minimum even value and its index of the said array of numbers: []
Flowchart:
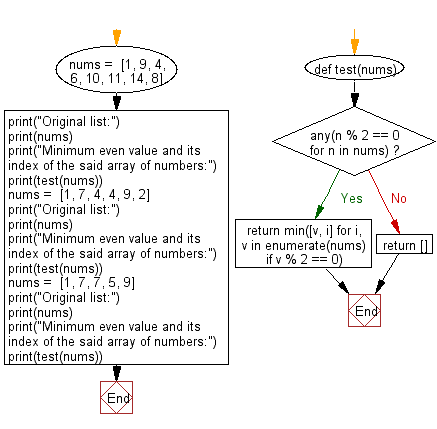
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find all even palindromes up to n.
Next: Filter for the numbers in a list whose sum of digits is >0, where the first digit can be negative..
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics