Python: Reverse the case of all strings. For those strings, which contain no letters, reverse the strings
Python Programming Puzzles: Exercise-52 with Solution
Write a Python program to reverse the case of all strings. For those strings, which contain no letters, reverse the strings.
Input: ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique'] Output: ['CAT', 'CATATATATCTSA', 'ABCDEFHIJKLMNOP', '521581932952421', '', 'FOO', 'UNIQUE'] Input: ['Green', 'Red', 'Orange', 'Yellow', '', 'White'] Output: ['gREEN', 'rED', 'oRANGE', 'yELLOW', '', 'wHITE'] Input: ['Hello', '!@#', '!@#$', '123#@!'] Output: ['hELLO', '!@#', '!@#$', '123#@!']
Visual Presentation:
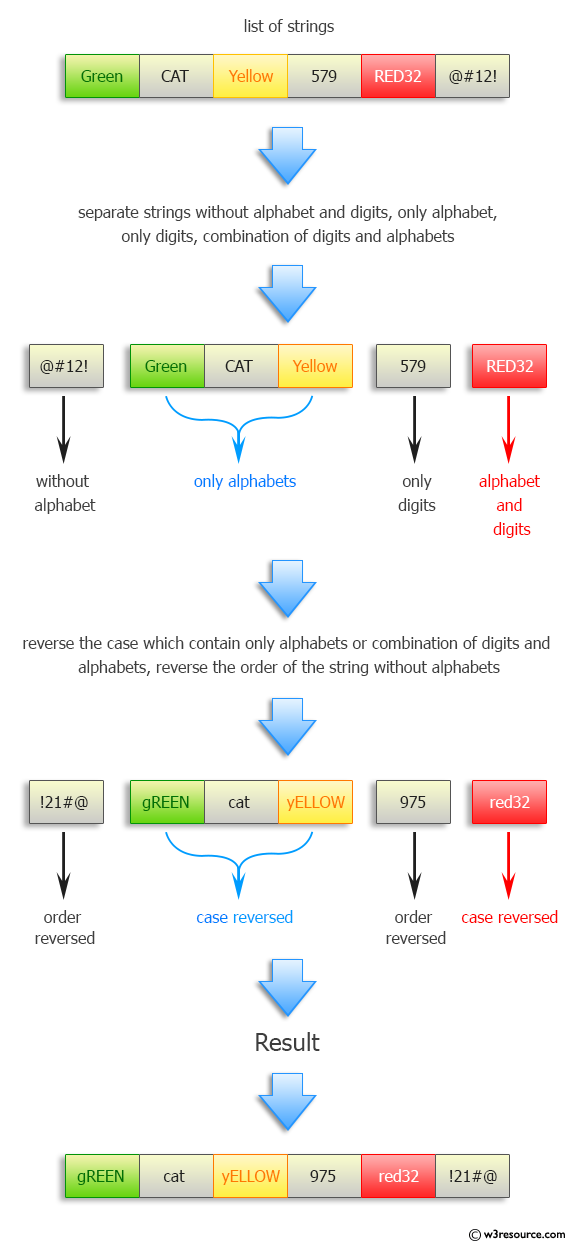
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of strings as input and returns a modified list
def test(strs: list[str]) -> list[str]:
# Use a list comprehension to create a new list with modified strings
# If a string contains any alphabetic characters, reverse its case; otherwise, reverse the string
return [s.swapcase() if any(c.isalpha() for c in s) else s[::-1] for s in strs]
# Create a list of strings named 'strs'
strs = ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique']
# Print a message indicating the task and the original list
print("Original list:")
# Print the original list of strings
print(strs)
# Print a message indicating the task and the result of the test function applied to 'strs'
print("Reverse the case of all strings. For those strings which contain no letters, reverse the strings:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Create another list of strings named 'strs'
strs = ['Green', 'Red', 'Orange', 'Yellow', '', 'White']
# Print a message indicating the task and the original list
print("\nOriginal list:")
# Print the original list of strings
print(strs)
# Print a message indicating the task and the result of the test function applied to 'strs'
print("Reverse the case of all strings. For those strings which contain no letters, reverse the strings:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Create yet another list of strings named 'strs'
strs = ["Hello", "!@#", "!@#$", "123#@!"]
# Print a message indicating the task and the original list
print("\nOriginal list:")
# Print the original list of strings
print(strs)
# Print a message indicating the task and the result of the test function applied to 'strs'
print("Reverse the case of all strings. For those strings which contain no letters, reverse the strings:")
# Print the result of the test function applied to 'strs'
print(test(strs))
Sample Output:
Original list: ['cat', 'catatatatctsa', 'abcdefhijklmnop', '124259239185125', '', 'foo', 'unique'] Reverse the case of all strings. For those strings which contain no letters, reverse the strings: ['CAT', 'CATATATATCTSA', 'ABCDEFHIJKLMNOP', '521581932952421', '', 'FOO', 'UNIQUE'] Original list: ['Green', 'Red', 'Orange', 'Yellow', '', 'White'] Reverse the case of all strings. For those strings which contain no letters, reverse the strings: ['gREEN', 'rED', 'oRANGE', 'yELLOW', '', 'wHITE'] Original list: ['Hello', '!@#', '!@#$', '123#@!'] Reverse the case of all strings. For those strings which contain no letters, reverse the strings: ['hELLO', '#@!', '$#@!', '!@#321']
Flowchart:
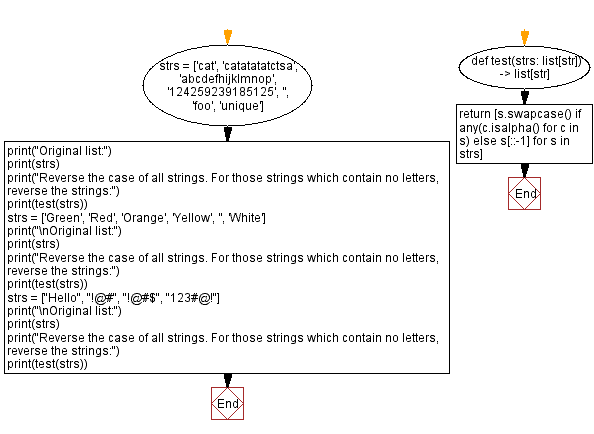
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the first n Fibonacci numbers.
Next: Find the product of the units digits in the numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-52.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics