Python: Biggest even number between two numbers inclusive
Python Programming Puzzles: Exercise-58 with Solution
Write a Python program to find the biggest even number between two numbers inclusive.
Input: m = 12 n = 51 Output: 50 Input: m = 1 n = 79 Output: 78 Input: m = 47 n = 53 Output: 52 Input: m = 100 n = 200 Output: 200
Visual Presentation:
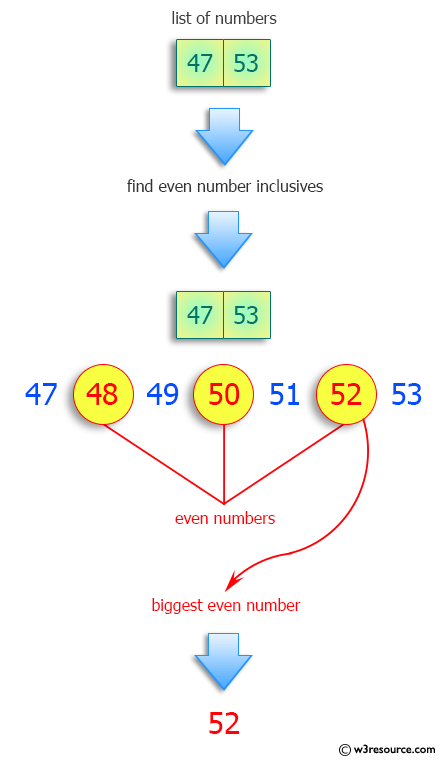
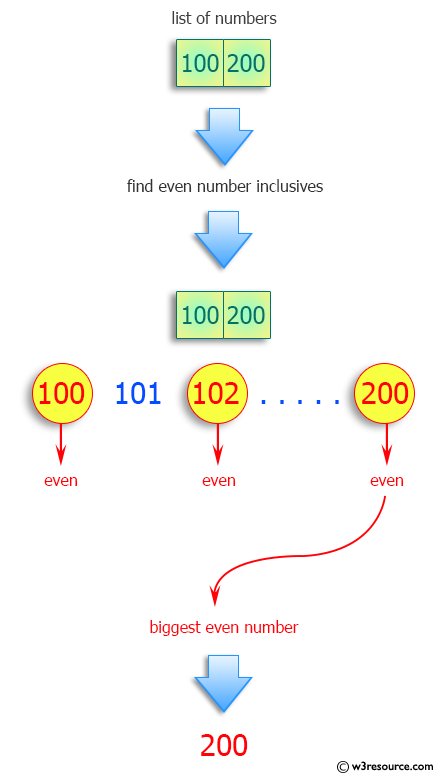
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes two parameters, m and n
def test(m, n):
# Check if m is greater than n or if both m and n are odd
if m > n or (m == n and m % 2 == 1):
# Return -1 if the condition is met
return -1
# Return n if n is even, otherwise return n - 1
return n if n % 2 == 0 else n - 1
# Set values for m and n
m = 12
n = 51
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the given values of m and n
print(test(m, n))
# Set new values for m and n
m = 1
n = 79
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the new values of m and n
print(test(m, n))
# Set another set of values for m and n
m = 47
n = 53
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the new values of m and n
print(test(m, n))
# Set additional values for m and n
m = 100
n = 200
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the additional values of m and n
print(test(m, n))
Sample Output:
Biggest even number between 12 and 51 50 Biggest even number between 1 and 79 78 Biggest even number between 47 and 53 52 Biggest even number between 100 and 200 200
Flowchart:
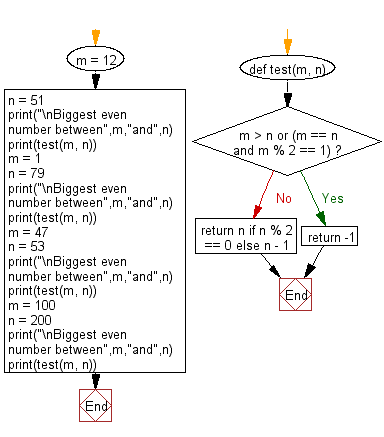
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes two parameters, p and q
def test(p, q):
# Initialize a variable 'n' with the value of 'q'
n = q
# Continue looping while the least significant bit of 'n' is 1 (n is odd)
while (n & 1) == 1:
# Decrement 'n' by 1
n -= 1
# Check if 'n' has become less than 'p'
if n < p:
# Return -1 if the condition is met
return -1
# Return the final value of 'n'
return n
# Set values for m and n
m = 12
n = 51
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the given values of m and n
print(test(m, n))
# Set new values for m and n
m = 1
n = 79
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the new values of m and n
print(test(m, n))
# Set another set of values for m and n
m = 47
n = 53
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the new values of m and n
print(test(m, n))
# Set additional values for m and n
m = 100
n = 200
# Print a message indicating the range of numbers
print("\nBiggest even number between", m, "and", n)
# Print the result of the test function applied to the additional values of m and n
print(test(m, n))
Sample Output:
Biggest even number between 12 and 51 50 Biggest even number between 1 and 79 78 Biggest even number between 47 and 53 52 Biggest even number between 100 and 200 200
Flowchart:
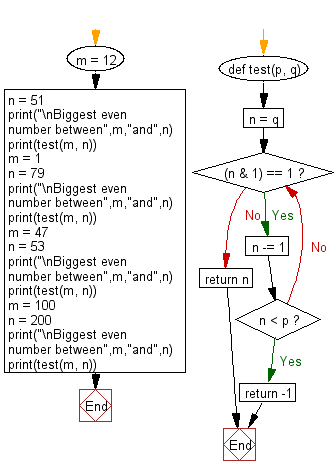
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sum of the magnitudes of the elements in the array with product signs.
Next: A valid filename.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-58.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics