Python: Find numbers that are adjacent to a prime number in the list, sorted without duplicates
Python Programming Puzzles: Exercise-60 with Solution
Prime number: A number that is divisible only by itself and 1 (e.g. 2, 3, 5, 7, 11).
Write a Python program to find a list of all numbers that are adjacent to a prime number in the list, sorted without duplicates.
Input: [2, 17, 16, 0, 6, 4, 5] Output: [2, 4, 6, 16, 17] Input: [1, 2, 19, 16, 6, 4, 10] Output: [1, 2, 16, 19] Input: [1, 2, 3, 5, 1, 16, 7, 11, 4] Output: [1, 2, 3, 4, 5, 7, 11, 16]
Visual Presentation:
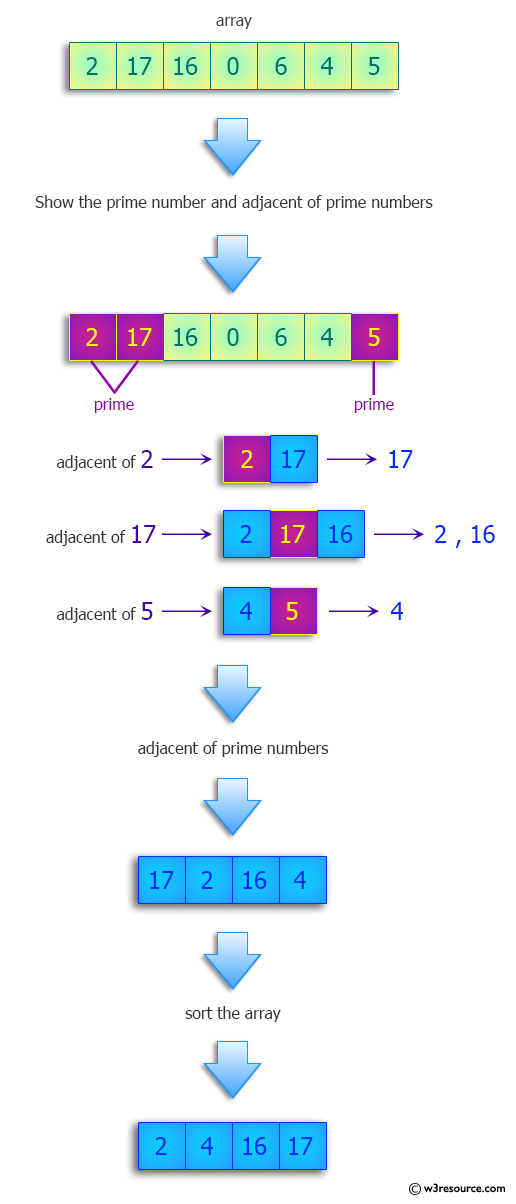
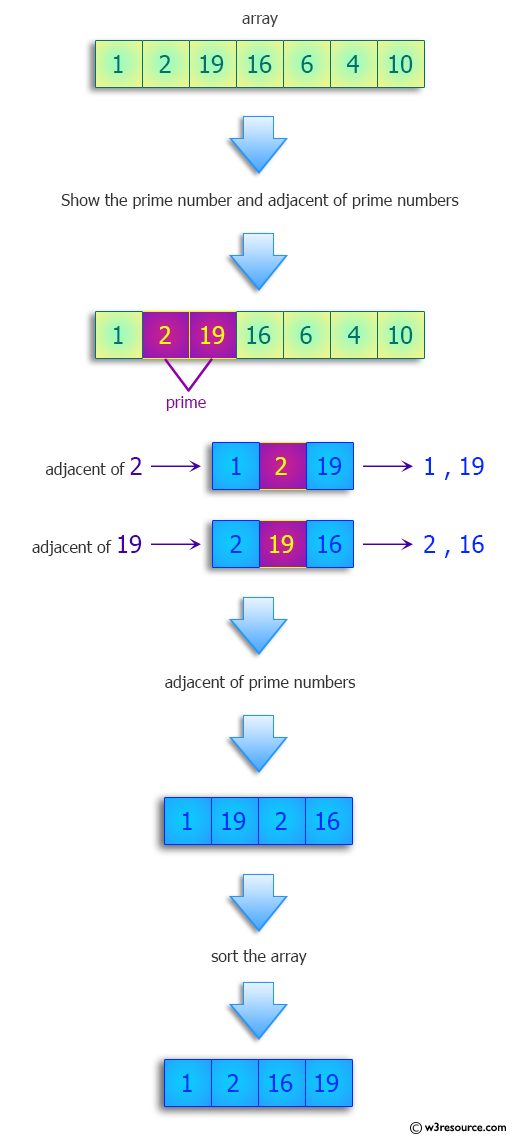
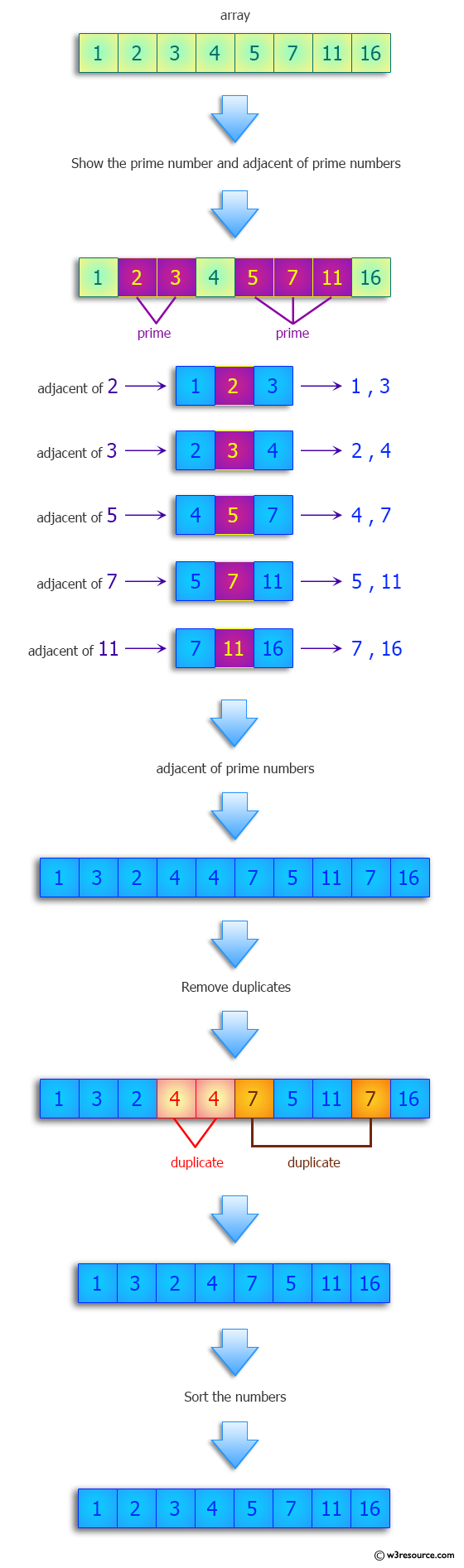
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Use a set comprehension to create a set of numbers that are adjacent to a prime number in the list
return sorted({
n for i, n in enumerate(nums)
if (i > 0 and prime(nums[i - 1])) or (i < len(nums) - 1 and prime(nums[i + 1]))
})
# Define a function named 'prime' that checks if a given number is prime
def prime(m):
# Check if the number is greater than 0 and is divisible by any number in the range (2, m - 1)
return all(m % i for i in range(2, m - 1))
# Set a list of numbers
nums = [2, 17, 16, 0, 6, 4, 5]
# Print a message indicating the original list of numbers
print("Original list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating numbers adjacent to a prime number, sorted without duplicates
print("Numbers that are adjacent to a prime number in the said list, sorted without duplicates:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
# Set another list of numbers
nums = [1, 2, 19, 16, 6, 4, 10]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating numbers adjacent to a prime number, sorted without duplicates
print("Numbers that are adjacent to a prime number in the said list, sorted without duplicates:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
# Set yet another list of numbers
nums = [1, 2, 3, 5, 1, 16, 7, 11, 4]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating numbers adjacent to a prime number, sorted without duplicates
print("Numbers that are adjacent to a prime number in the said list, sorted without duplicates:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
Sample Output:
Original list of numbers: [2, 17, 16, 0, 6, 4, 5] Numbers that are adjacent to a prime number in the said list, sorted without duplicates: [2, 4, 16, 17] Original list of numbers: [1, 2, 19, 16, 6, 4, 10] Numbers that are adjacent to a prime number in the said list, sorted without duplicates: [1, 2, 16, 19] Original list of numbers: [1, 2, 3, 5, 1, 16, 7, 11, 4] Numbers that are adjacent to a prime number in the said list, sorted without duplicates: [1, 2, 3, 4, 5, 7, 11, 16]
Flowchart:
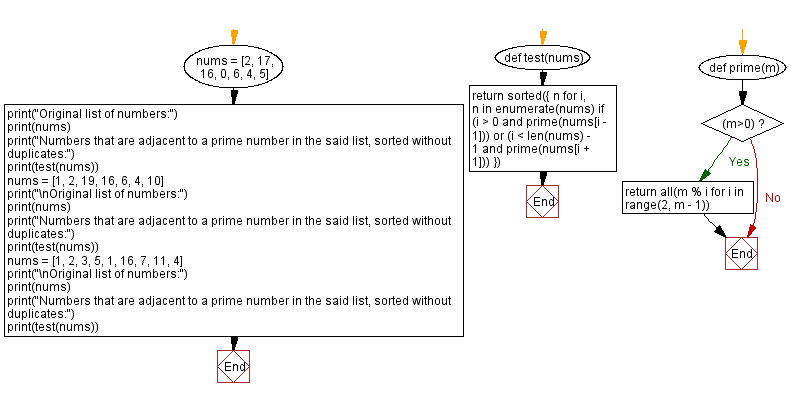
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: A valid filename.
Next: Find the number which when appended to the list makes the total 0.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-60.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics