Python: Find the dictionary key whose case is different than all other keys
Dictionary Key with Odd Case
Write a Python program to find the dictionary key whose case is different from all other keys.
Input: {'red': '', 'GREEN': '', 'blue': 'orange'} Output: GREEN Input: {'RED': '', 'GREEN': '', 'orange': '#125GD'} Output: orange
Visual Presentation:
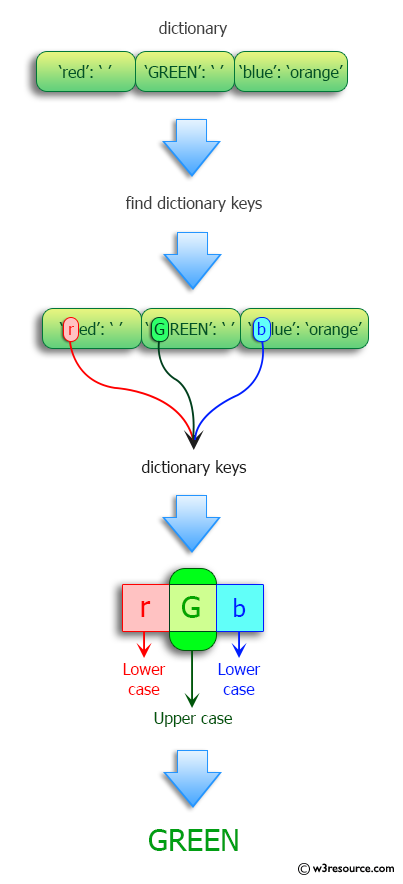
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
def test(dict_data):
# Iterate over each key in the dictionary
for different in dict_data:
# Check if the case of the current key is different from all other keys
if all(k.islower() != different.islower() for k in dict_data if k != different):
# Return the key with a different case
return different
# Example 1
dict_data1 = {"red": "", "GREEN": "", "blue": "orange"}
print("Original dictionary key-values:")
print(dict_data1)
print("Find the dictionary key whose case is different than all other keys:")
print(test(dict_data1))
# Example 2
dict_data2 = {"RED": "", "GREEN": "", "orange": "#125GD"}
print("\nOriginal dictionary key-values:")
print(dict_data2)
print("Find the dictionary key whose case is different than all other keys:")
print(test(dict_data2))
Sample Output:
Original dictionary key-values: {'red': '', 'GREEN': '', 'blue': 'orange'} Find the dictionary key whose case is different than all other keys: GREEN Original dictionary key-values: {'RED': '', 'GREEN': '', 'orange': '#125GD'} Find the dictionary key whose case is different than all other keys: orange
Flowchart:
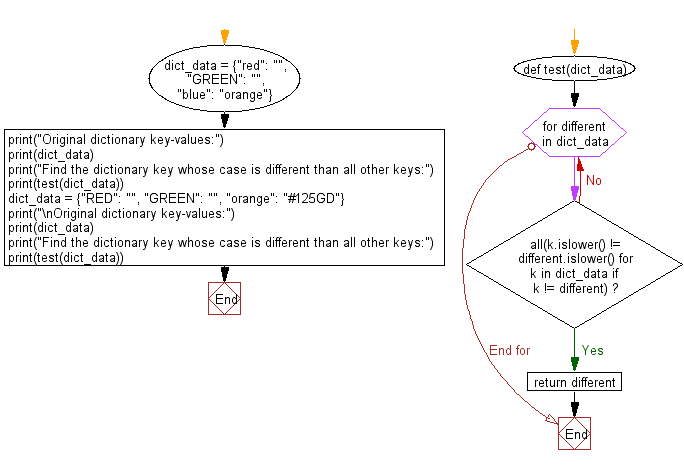
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the number which when appended to the list makes the total 0.
Next: Find the sum of the even elements that are at odd indices in a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics