Python: Find the sum of the even elements that are at odd indices
Sum of Even Elements at Odd Indices
Write a Python program to find the sum of the even elements that are at odd indices in a given list.
Input: [1, 2, 3, 4, 5, 6, 7] Output: 12 Input: [1, 2, 8, 3, 9, 4] Output: 6
Visual Presentation:
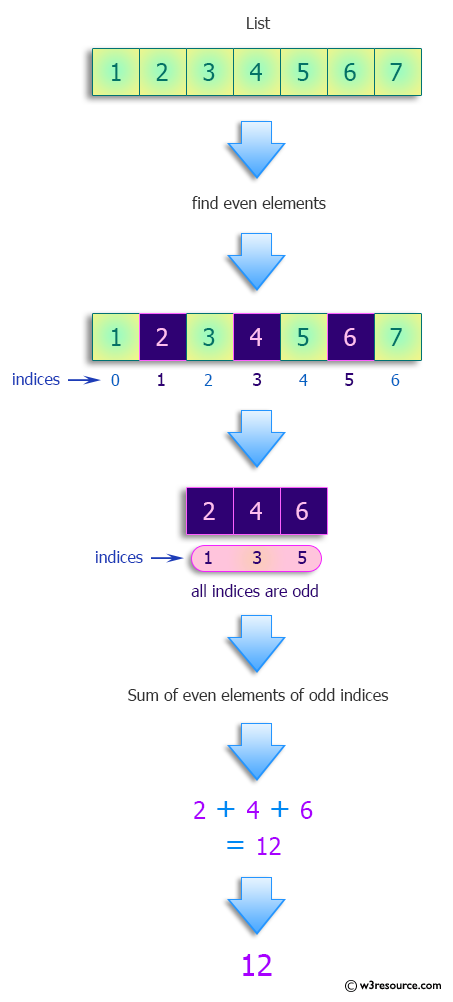
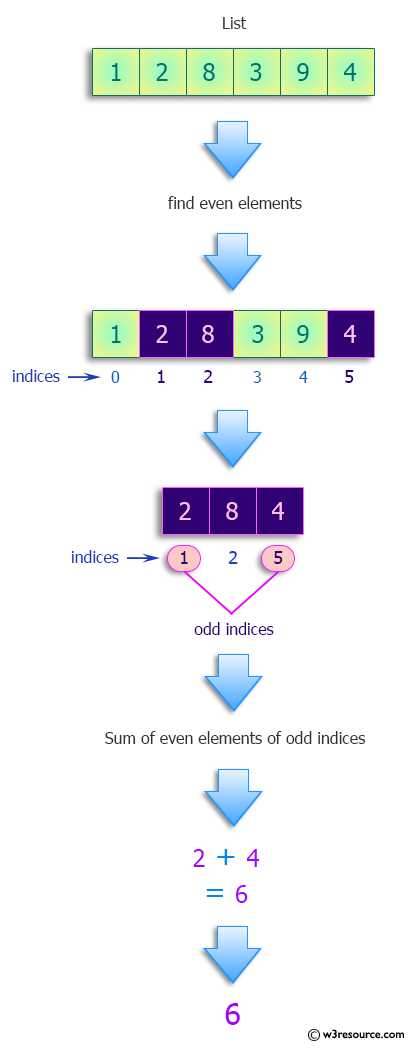
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
def test(nums):
# Sum the even elements at odd indices using list slicing and a conditional expression
return sum(i for i in nums[1::2] if i % 2 == 0)
# Example 1
nums1 = [1, 2, 3, 4, 5, 6, 7]
print("Original list of numbers:")
print(nums1)
print("Sum of the even elements of the said list that are at odd indices:")
print(test(nums1))
# Example 2
nums2 = [1, 2, 8, 3, 9, 4]
print("\nOriginal list of numbers:")
print(nums2)
print("Sum of the even elements of the said list that are at odd indices:")
print(test(nums2))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 6, 7] Sum of the even elements of the said list that are at odd indices: 12 Original list of numbers: [1, 2, 8, 3, 9, 4] Sum of the even elements of the said list that are at odd indices: 6
Flowchart:
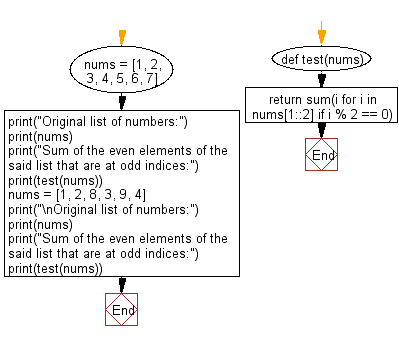
Sample Solution-2:
Python Code:
def test(nums):
# Sum the even elements at odd indices using a list comprehension
return sum([nums[i] for i in range(len(nums)) if i % 2 == 1 and nums[i] % 2 == 0])
# Example 1
nums1 = [1, 2, 3, 4, 5, 6, 7]
print("Original list of numbers:")
print(nums1)
print("Sum of the even elements of the said list that are at odd indices:")
print(test(nums1))
# Example 2
nums2 = [1, 2, 8, 3, 9, 4]
print("\nOriginal list of numbers:")
print(nums2)
print("Sum of the even elements of the said list that are at odd indices:")
print(test(nums2))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 6, 7] Sum of the even elements of the said list that are at odd indices: 12 Original list of numbers: [1, 2, 8, 3, 9, 4] Sum of the even elements of the said list that are at odd indices: 6
Flowchart:
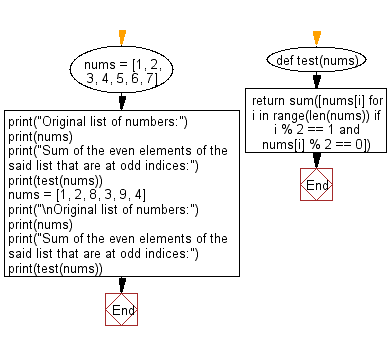
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the dictionary key whose case is different than all other keys.
Next: Find the string consisting of all the words whose lengths are prime numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics