Python: Circular shift number
Circular Shift of Digits
Write a Python program to shift the decimal digits n places to the left, wrapping the extra digits around. If the shift > the number of digits in n, reverse the string.
Input: n = 12345 and shift = 1 Output: Result = 23451 Input: n = 12345 and shift = 2 Output: Result = 34512 Input: n = 12345 and shift = 3 Output: Result = 45123 Input: n = 12345 and shift = 5 Output: Result = 12345 Input: n = 12345 and shift = 6 Output: Result = 54321
Visual Presentation:
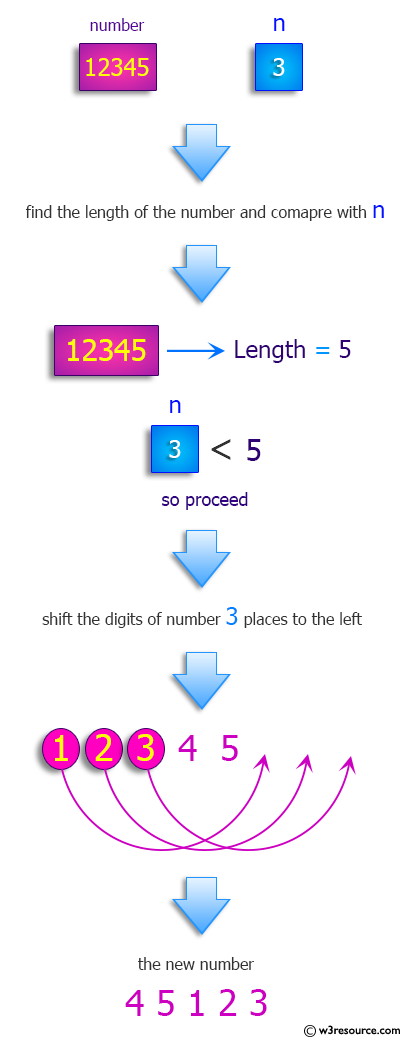
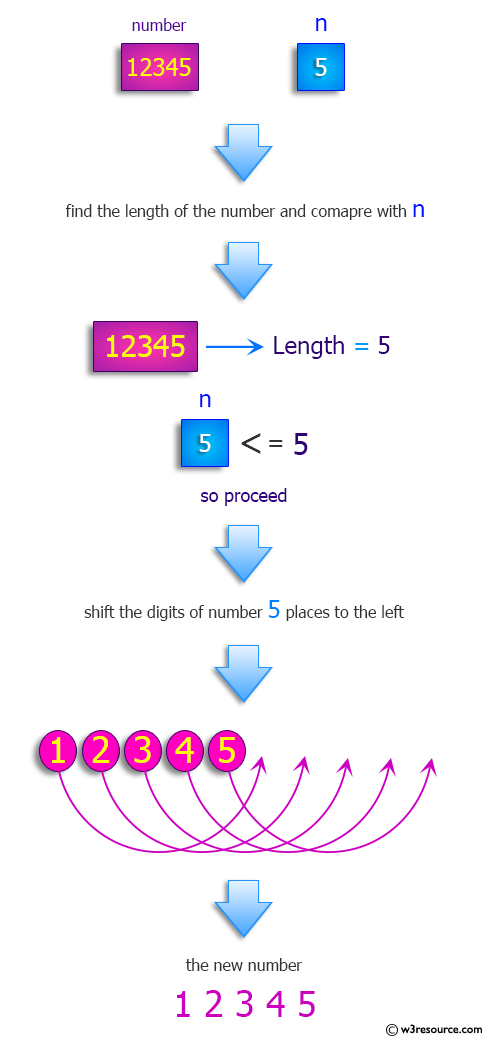
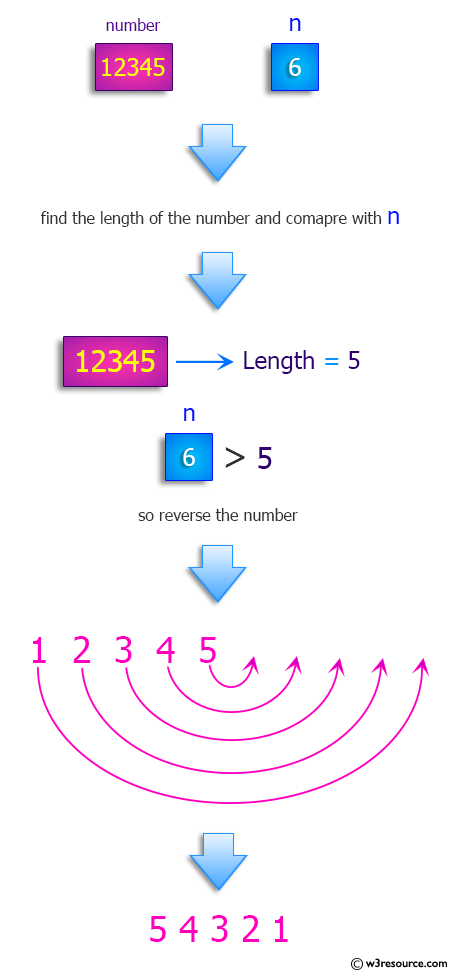
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
def test(n, shift):
# Convert the number to a string
s = str(n)
# Check if shift is greater than the number of digits in n
if shift > len(s):
# If so, reverse the string
return s[::-1]
# Shift the decimal digits to the left by 'shift' places
return s[shift:] + s[:shift]
# Display the purpose of the code
print("Shift the decimal digits n places to the left. If shift > the number of digits of n, reverse the string.")
# Example 1
n1 = 12345
shift1 = 1
print("\nn =", n1, " and shift =", shift1)
print("Result =", test(n1, shift1))
# Example 2
n2 = 12345
shift2 = 2
print("\nn =", n2, " and shift =", shift2)
print("Result =", test(n2, shift2))
# Example 3
n3 = 12345
shift3 = 3
print("\nn =", n3, " and shift =", shift3)
print("Result =", test(n3, shift3))
# Example 4
n4 = 12345
shift4 = 5
print("\nn =", n4, " and shift =", shift4)
print("Result =", test(n4, shift4))
# Example 5
n5 = 12345
shift5 = 6
print("\nn =", n5, " and shift =",shift5)
print("Result = ",test(n5, shift))
Sample Output:
Shift the decimal digits n places to the left. If shift > the number of digits of n, reverse the string.: n = 12345 and shift = 1 Result = 23451 n = 12345 and shift = 2 Result = 34512 n = 12345 and shift = 3 Result = 45123 n = 12345 and shift = 5 Result = 12345 n = 12345 and shift = 6 Result = 54321
Flowchart:
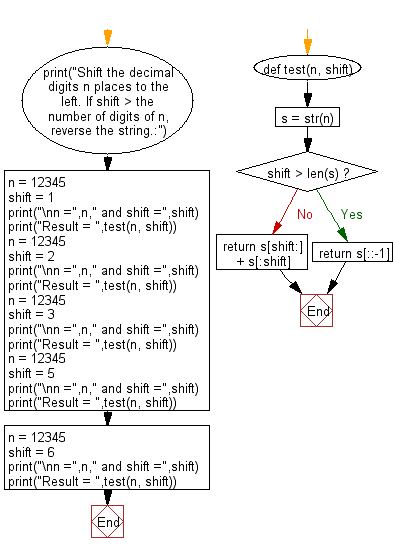
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
def test(n, shift):
# Convert the number to a list of individual digits
shifted_digits = [int(x) for x in str(n)]
# Shift the digits to the left by 'shift' places using list manipulation
for i in range(shift):
shifted_digits.append(shifted_digits.pop(0))
# Check if shift is greater than the number of digits in n
if shift > len(shifted_digits):
# If so, reverse the string representation of n
return str(n)[::-1]
else:
# Convert the shifted digits back to a string and join them
return ''.join(str(x) for x in shifted_digits)
# Display the purpose of the code
print("Shift the decimal digits n places to the left. If shift > the number of digits of n, reverse the string.")
# Example 1
n1 = 12345
shift1 = 1
print("\nn =", n1, " and shift =", shift1)
print("Result =", test(n1, shift1))
# Example 2
n2 = 12345
shift2 = 2
print("\nn =", n2, " and shift =", shift2)
print("Result =", test(n2, shift2))
# Example 3
n3 = 12345
shift3 = 3
print("\nn =", n3, " and shift =", shift3)
print("Result =", test(n3, shift3))
# Example 4
n4 = 12345
shift4 = 5
print("\nn =", n4, " and shift =", shift4)
print("Result =", test(n4, shift4))
# Example 5
n5 = 12345
shift5 = 6
print("\nn =", n5, " and shift =", shift5)
print("Result =", test(n5, shift5))
Sample Output:
Shift the decimal digits n places to the left. If shift > the number of digits of n, reverse the string.: n = 12345 and shift = 1 Result = 23451 n = 12345 and shift = 2 Result = 34512 n = 12345 and shift = 3 Result = 45123 n = 12345 and shift = 5 Result = 12345 n = 12345 and shift = 6 Result = 54321
Flowchart:
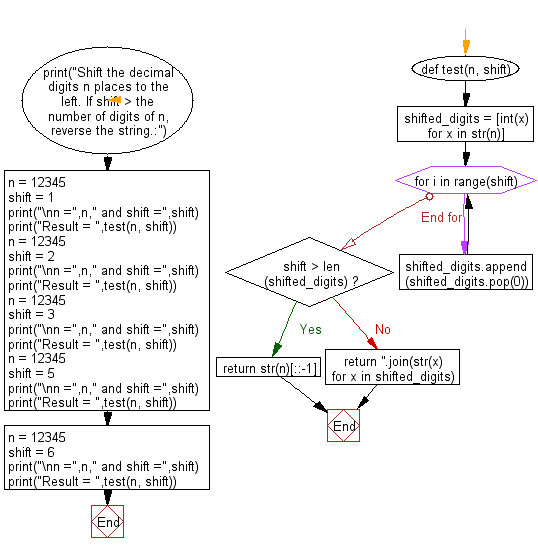
For more Practice: Solve these Related Problems:
- Write a Python program to perform a circular left shift of the digits in an integer by a given number of places.
- Write a Python program to convert an integer to a string, rotate its characters by a specified shift value, and convert it back.
- Write a Python program to implement circular digit rotation such that if the shift exceeds the number of digits, the string is reversed.
- Write a Python program to perform a circular left rotation on an integer’s digits, handling edge cases where the shift equals the digit count.
Go to:
Previous: Find the string consisting of all the words whose lengths are prime numbers.
Next: Find the indices of the closest pair from given a list of numbers.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.