Python: Find a string which, when each character is shifted (ASCII incremented) by shift
ASCII Shift for String Transformation
Write a Python program to find a string which, when each character is shifted (ASCII incremented) by shift, gives the result.
Input: Ascii character table Shift = 1 Output: @rbhhbg`q`bsdqs`akd Input: Ascii character table Shift = -1 Output: Btdjj!dibsbdufs!ubcmf
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
def test(strs, shift):
# Joining characters after shifting them by the specified amount
return "".join(chr(ord(c) - shift) for c in strs)
# Example 1
strs1 = "Ascii character table"
print("Original string:")
print(strs1)
shift1 = 1
print('Shift =', shift1)
print("A new string which, when each character is shifted (ASCII incremented) by shift in the said string:")
print(test(strs1, shift1))
# Example 2
strs2 = "Ascii character table"
print("\nOriginal string:")
print(strs2)
shift2 = -1
print('Shift =', shift2)
print("A new string which, when each character is shifted (ASCII incremented) by shift in the said string:")
print(test(strs2, shift2))
Sample Output:
Original string: Ascii character table Shift = 1 A new string which, when each character is shifted (ASCII incremented) by shift in the said string: @rbhhbg`q`bsdqs`akd Original string: Ascii character table Shift = -1 A new string which, when each character is shifted (ASCII incremented) by shift in the said string: Btdjj!dibsbdufs!ubcmf
Flowchart:
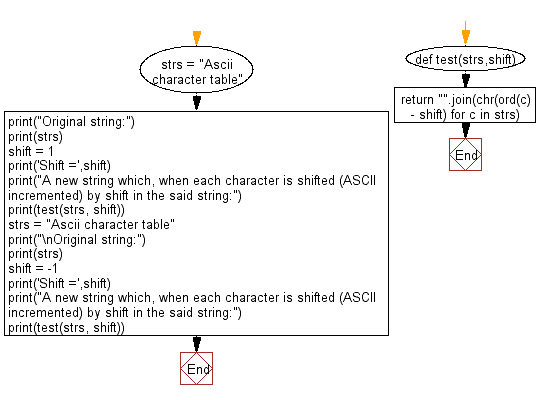
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
def test(strs, shift):
# Using list comprehension to generate a list of characters after shifting
shifted_chars = [chr(ord(strs[i]) - shift) for i in range(len(strs))]
# Joining the characters to form the final shifted string
return "".join(shifted_chars)
# Example 1
strs1 = "Ascii character table"
print("Original string:")
print(strs1)
shift1 = 1
print('Shift =', shift1)
print("A new string which, when each character is shifted (ASCII decremented) by shift in the said string:")
print(test(strs1, shift1))
# Example 2
strs2 = "Ascii character table"
print("\nOriginal string:")
print(strs2)
shift2 = -1
print('Shift =', shift2)
print("A new string which, when each character is shifted (ASCII decremented) by shift in the said string:")
print(test(strs2, shift2))
Sample Output:
Original string: Ascii character table Shift = 1 A new string which, when each character is shifted (ASCII incremented) by shift in the said string: @rbhhbg`q`bsdqs`akd Original string: Ascii character table Shift = -1 A new string which, when each character is shifted (ASCII incremented) by shift in the said string: Btdjj!dibsbdufs!ubcmf
Flowchart:
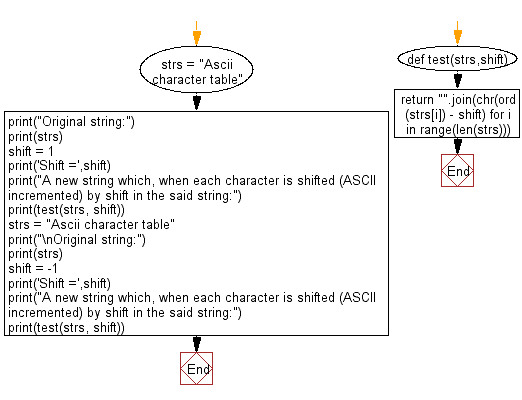
For more Practice: Solve these Related Problems:
- Write a Python program to transform a string by shifting each character’s ASCII value by a given offset and output the resulting string.
- Write a Python program to implement an ASCII shift cipher that increments each character’s value by a specified amount.
- Write a Python program to modify a string by applying an ASCII shift to each character and handling wrap-around for alphabets.
- Write a Python program to create a function that shifts a string’s characters by a given integer and returns the transformed string.
Go to:
Previous: Find the indices of the closest pair from given a list of numbers.
Next: Find all 5's in integers less than n that are divisible by 9 or 15.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.