Python: Find all 5's in integers less than n that are divisible by 9 or 15
Fives Divisible by 9 or 15
Write a Python program to find all 5's in integers less than n that are divisible by 9 or 15.
Input: Value of n = 50 Output: [[15, 1], [45, 1]] Input: Value of n = 65 Output: [[15, 1], [45, 1], [54, 0]] Input: Value of n = 75 Output: [[15, 1], [45, 1], [54, 0]] Input: Value of n = 85 Output: [[15, 1], [45, 1], [54, 0], [75, 1]] Input: Value of n = 150 Output: [[15, 1], [45, 1], [54, 0], [75, 1], [105, 2], [135, 2]]
Visual Presentation:
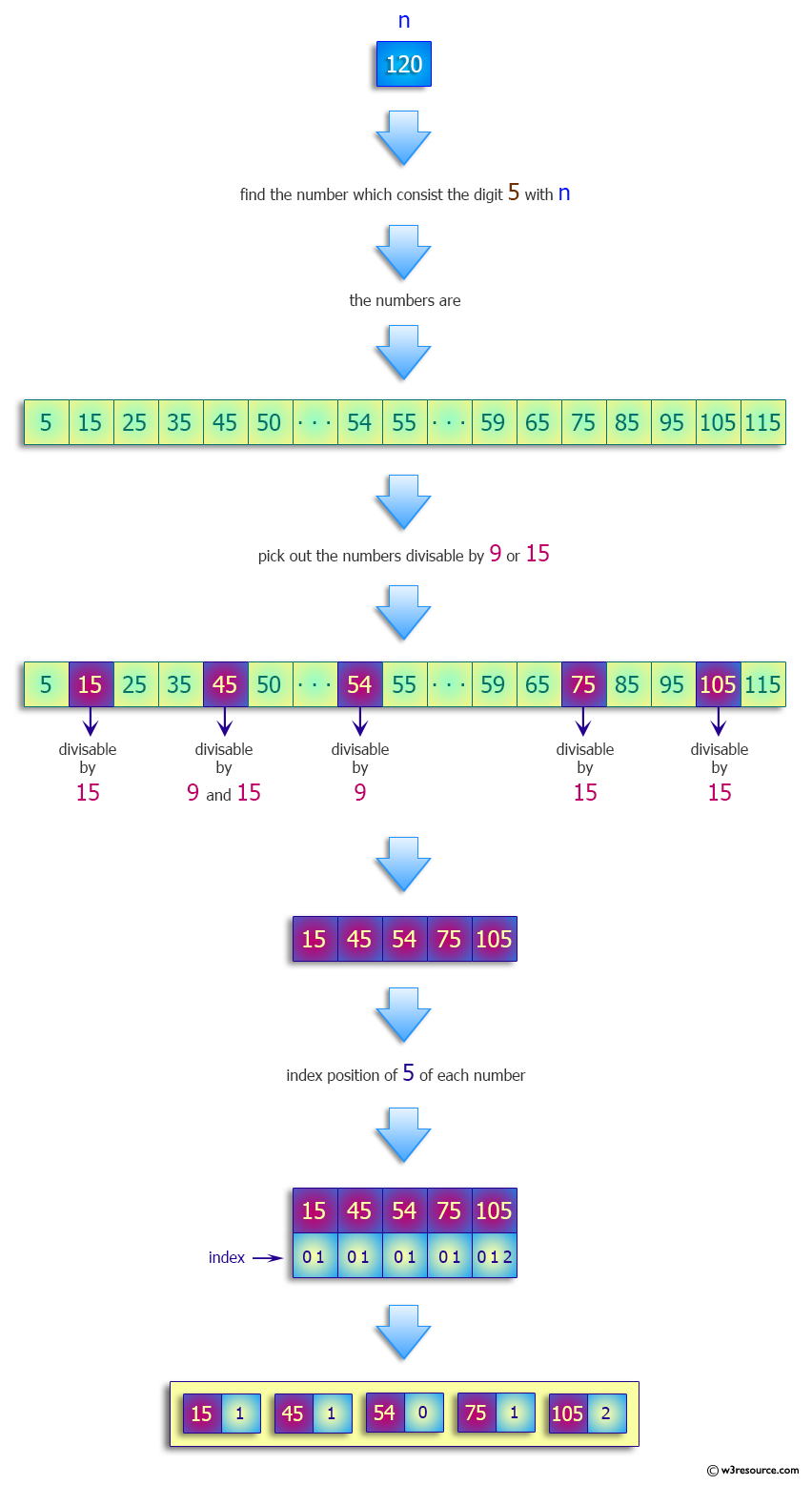
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes an integer 'n' as input
def test(n):
# Using list comprehension to generate a list of pairs [i, j] for each i and j satisfying the conditions
return [[i, j] for i in range(n) for j in range(len(str(i))) if str(i)[j] == '5' and (i % 15 == 0 or i % 9 == 0)]
# Example 1
n1 = 50
print("Value of n =", n1)
print("5's in integers less than", n1, "that are divisible by 9 or 15:")
print(test(n1))
# Example 2
n2 = 65
print("\nValue of n =", n2)
print("5's in integers less than", n2, "that are divisible by 9 or 15:")
print(test(n2))
# Example 3
n3 = 75
print("\nValue of n =", n3)
print("5's in integers less than", n3, "that are divisible by 9 or 15:")
print(test(n3))
# Example 4
n4 = 85
print("\nValue of n =", n4)
print("5's in integers less than", n4, "that are divisible by 9 or 15:")
print(test(n4))
# Example 5
n5 = 150
print("\nValue of n =", n5)
print("5's in integers less than", n5, "that are divisible by 9 or 15:")
print(test(n5))
Sample Output:
Value of n = 50 5's in integers less than 50 that are divisible by 9 or 15: [[15, 1], [45, 1]] Value of n = 65 5's in integers less than 65 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0]] Value of n = 75 5's in integers less than 75 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0]] Value of n = 85 5's in integers less than 85 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0], [75, 1]] Value of n = 150 5's in integers less than 150 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0], [75, 1], [105, 2], [135, 2]]
Flowchart:
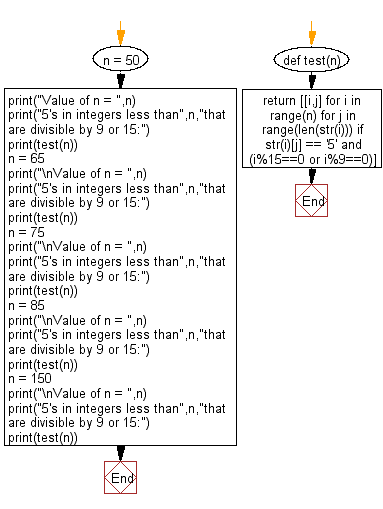
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes an integer 'n' as input
def test(n):
# Using list comprehension to generate a list of pairs [i, j] for each i and j satisfying the conditions
return [[i, j] for i in range(n) if (i % 9 == 0 or i % 15 == 0) for j, c in enumerate(str(i)) if c == '5']
# Example 1
n1 = 50
print("Value of n =", n1)
print("5's in integers less than", n1, "that are divisible by 9 or 15:")
print(test(n1))
# Example 2
n2 = 65
print("\nValue of n =", n2)
print("5's in integers less than", n2, "that are divisible by 9 or 15:")
print(test(n2))
# Example 3
n3 = 75
print("\nValue of n =", n3)
print("5's in integers less than", n3, "that are divisible by 9 or 15:")
print(test(n3))
# Example 4
n4 = 85
print("\nValue of n =", n4)
print("5's in integers less than", n4, "that are divisible by 9 or 15:")
print(test(n4))
# Example 5
n5 = 150
print("\nValue of n =", n5)
print("5's in integers less than", n5, "that are divisible by 9 or 15:")
print(test(n5))
Sample Output:
Value of n = 50 5's in integers less than 50 that are divisible by 9 or 15: [[15, 1], [45, 1]] Value of n = 65 5's in integers less than 65 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0]] Value of n = 75 5's in integers less than 75 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0]] Value of n = 85 5's in integers less than 85 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0], [75, 1]] Value of n = 150 5's in integers less than 150 that are divisible by 9 or 15: [[15, 1], [45, 1], [54, 0], [75, 1], [105, 2], [135, 2]]
Flowchart:
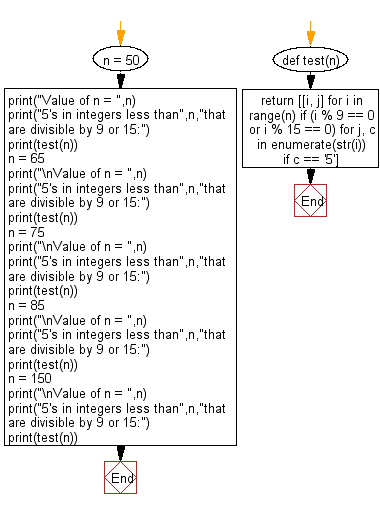
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find a string which, when each character is shifted (ASCII incremented) by shift.
Next: Create a new string by taking s, and word by word rearranging its characters in ASCII order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics