Python: Create a new string by taking a string, and word by word rearranging its characters in ASCII order
Rearrange Words by ASCII
Write a Python program to create a new string by taking a string, and word by word rearranging its characters in ASCII order.
Input: Ascii character table Output: Aciis aaccehrrt abelt Input: maltos won Output: almost now
Visual Presentation:
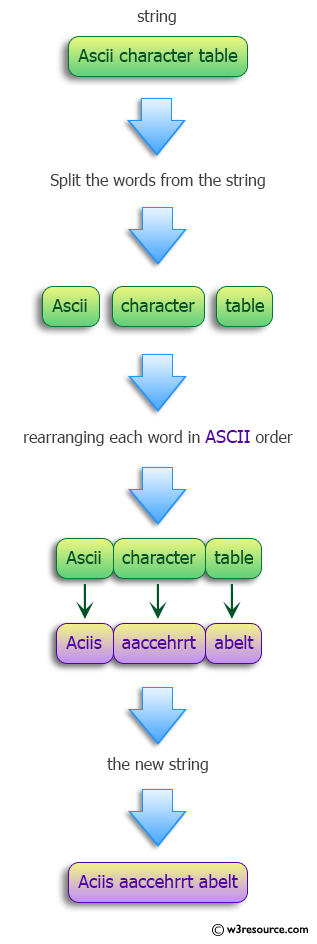
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Using list comprehension to iterate over words in the input string, sorting the characters of each word in ASCII order
# Then, joining the sorted words into a new string with spaces between them
return " ".join("".join(sorted(w)) for w in strs.split(' '))
# Example 1
strs1 = "Ascii character table"
print("Original string:", strs1)
print("New string by said string, and word by word rearranging its characters in ASCII order:")
print(test(strs1))
# Example 2
strs2 = "maltos won"
print("\nOriginal string:", strs2)
print("New string by said string, and word by word rearranging its characters in ASCII order:")
print(test(strs2))
Sample Output:
Original string: Ascii character table New string by said string, and word by word rearranging its characters in ASCII order: Aciis aaccehrrt abelt Original string: maltos won New string by said string, and word by word rearranging its characters in ASCII order: almost now
Flowchart:
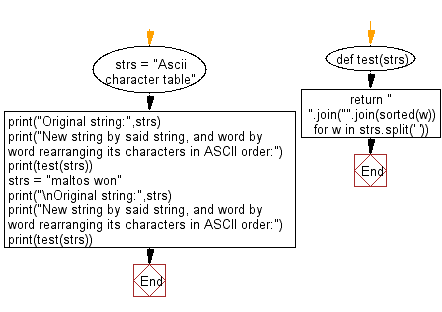
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Split the input string into a list of words
words = strs.split(' ')
rwords = [] # Initialize an empty list to store the results
# Iterate over each word in the list of words
for word in words:
occurrences = {} # Initialize an empty dictionary to store character occurrences
# Count occurrences of each character in the word
for c in word:
occurrences[c] = occurrences.get(c, 0) + 1
subsequence = [] # Initialize an empty list to store characters in the subsequence
# Iterate over the dictionary items and create a subsequence based on character occurrences
for c, count in occurrences.items():
subsequence += [c]*count
subsequence.sort() # Sort the subsequence in ASCII order
# Repeat the subsequence to match the length of the original word
subsequence = subsequence*(len(word)//len(subsequence)) + subsequence[:len(word)%len(subsequence)]
# Join the characters of the subsequence to form a rearranged word and append it to the result list
rwords.append(''.join(subsequence))
# Join the rearranged words into a new string with spaces between them
return ' '.join(rwords)
# Example 1
strs1 = "Ascii character table"
print("Original string:", strs1)
print("New string by said string, and word by word rearranging its characters in ASCII order:")
print(test(strs1))
# Example 2
strs2 = "maltos won"
print("\nOriginal string:", strs2)
print("New string by said string, and word by word rearranging its characters in ASCII order:")
print(test(strs2))
Output:
Original string: Ascii character table New string by said string, and word by word rearranging its characters in ASCII order: Aciis aaccehrrt abelt Original string: maltos won New string by said string, and word by word rearranging its characters in ASCII order: almost now
Flowchart:
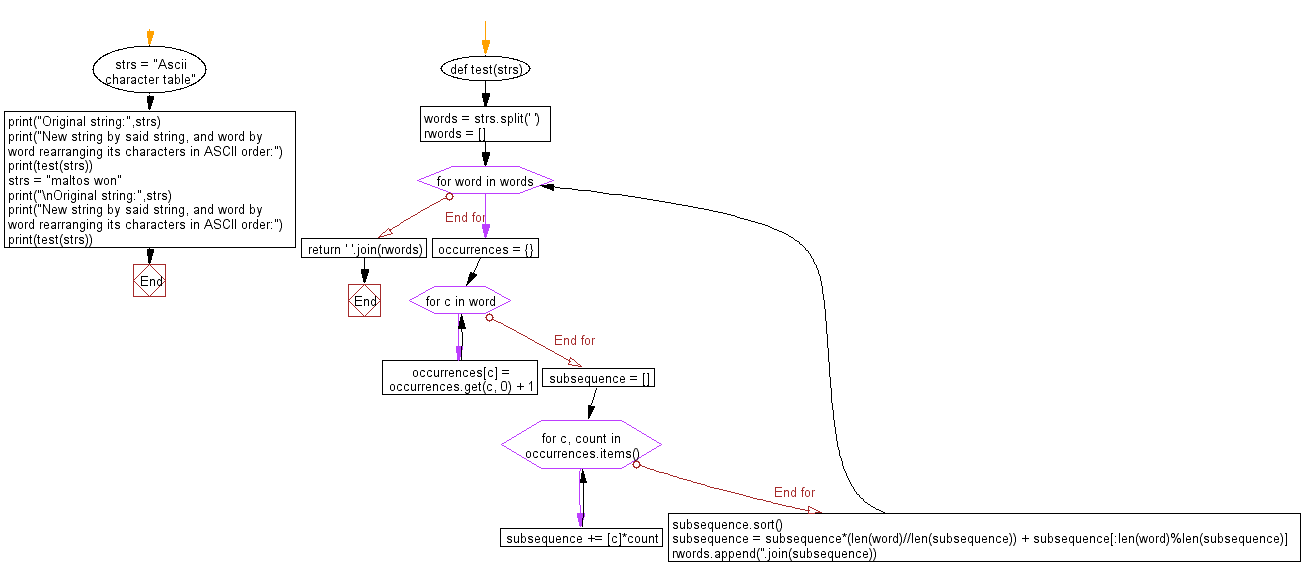
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find all 5's in integers less than n that are divisible by 9 or 15.
Next: Find the first negative balance.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics