Python: Find the two closest distinct numbers in a given a list of numbers
Closest Distinct Pair in List
Write a Python program to find the two closest distinct numbers in a given list of numbers.
Input: [1.3, 5.24, 0.89, 21.0, 5.27, 1.3] Output: [5.24, 5.27] Input: [12.02, 20.3, 15.0, 19.0, 11.0, 14.99, 17.0, 17.0, 14.4, 16.8] Output: [14.99, 15.0]
Visual Presentation:
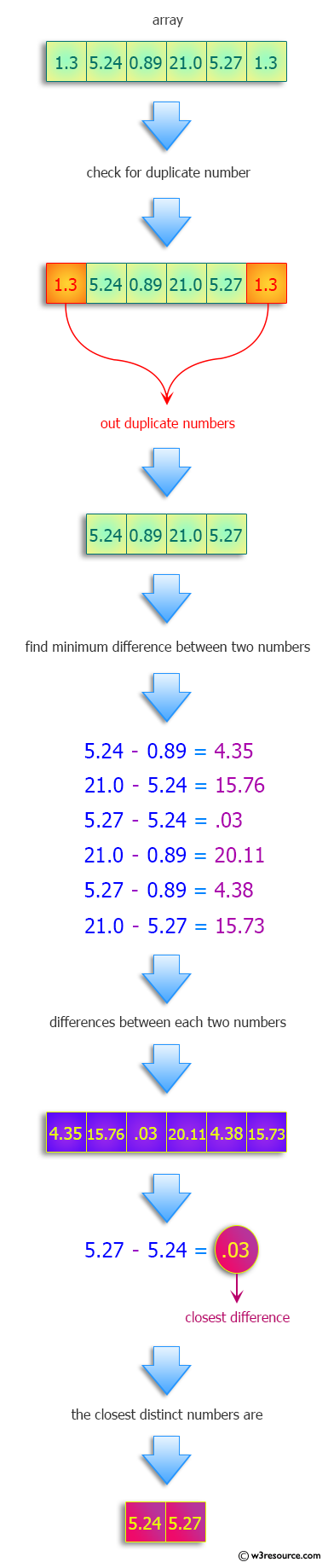
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Sort the unique elements in the list in ascending order
s = sorted(set(nums))
# Use list comprehension to find pairs of adjacent elements
# Then, find the pair with the smallest difference
return min([[a, b] for a, b in zip(s, s[1:])], key=lambda x: x[1] - x[0])
# Example 1
nums1 = [1.3, 5.24, 0.89, 21.0, 5.27, 1.3]
print("List of numbers:", nums1)
print("Two closest distinct numbers in the said list of numbers:")
print(test(nums1))
# Example 2
nums2 = [12.02, 20.3, 15.0, 19.0, 11.0, 14.99, 17.0, 17.0, 14.4, 16.8]
print("\nList of numbers:", nums2)
print("Two closest distinct numbers in the said list of numbers:")
print(test(nums2))
Sample Output:
List of numbers: [1.3, 5.24, 0.89, 21.0, 5.27, 1.3] Two closest distinct numbers in the said list of numbers: [5.24, 5.27] List of numbers: [12.02, 20.3, 15.0, 19.0, 11.0, 14.99, 17.0, 17.0, 14.4, 16.8] Two closest distinct numbers in the said list of numbers: [14.99, 15.0]
Flowchart:
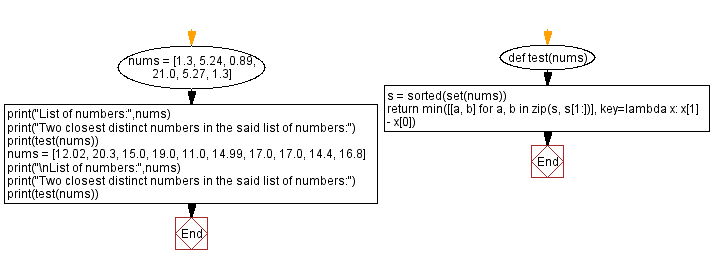
For more Practice: Solve these Related Problems:
- Write a Python program to find two distinct numbers in a list that have the smallest absolute difference and return them.
- Write a Python program to sort a list and then identify the pair of distinct numbers with the minimum gap, returning their values.
- Write a Python program to use a two-pointer technique on a sorted list to detect the closest distinct pair and then map back their original indices.
- Write a Python program to iterate through a list and compare adjacent differences to find the closest pair of different numbers.
Go to:
Previous: Convert GPAs to letter grades.
Next: Find the largest negative and smallest positive numbers.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.