Python: Split a string of words separated by commas and spaces into 2 lists: words and separators
Python Programming Puzzles: Exercise-8 with Solution
Write a Python program to split a string of words separated by commas and spaces into two lists, words and separators.
Input: W3resource Python, Exercises. Output: [['W3resource', 'Python', 'Exercises.'], [' ', ', ']] Input: The dance, held in the school gym, ended at midnight. Output:
[['The', 'dance', 'held', 'in', 'the', 'school', 'gym', 'ended', 'at', 'midnight.'], [' ', ', ', ' ', ' ', ' ', ' ', ', ', ' ', ' ']] Input: The colors in my studyroom are blue, green, and yellow. Output: [['The', 'colors', 'in', 'my', 'studyroom', 'are', 'blue', 'green', 'and', 'yellow.'], [' ', ' ', ' ', ' ', ' ', ' ', ', ', ', ', ' ']]
Visual Presentation:
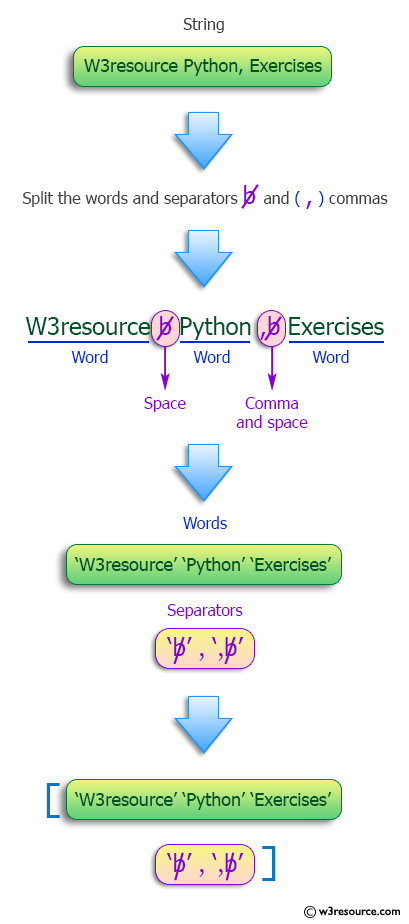
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'string' as input
def test(string):
# Import the 're' module for regular expressions
import re
# Use regular expression to split the string into words and separators and store in the 'merged' list
merged = re.split(r"([ ,]+)", string)
# Return a list containing two sublists: words (even indices) and separators (odd indices) from the 'merged' list
return [merged[::2], merged[1::2]]
# Assign a specific string 's' to the variable
s = "W3resource Python, Exercises."
# Print the original string
print("Original string:", s)
# Print a message indicating the operation to be performed on the string
print("Split the said string into 2 lists: words and separators:")
# Print the result of the test function applied to the string 's'
print(test(s))
# Assign a different string 's' to the variable
s = "The dance, held in the school gym, ended at midnight."
# Print the original string
print("\nOriginal string:", s)
# Print a message indicating the operation to be performed on the string
print("Split the said string into 2 lists: words and separators:")
# Print the result of the test function applied to the modified string 's'
print(test(s))
# Assign another string 's' to the variable
s = "The colors in my studyroom are blue, green, and yellow."
# Print the original string
print("\nOriginal string:", s)
# Print a message indicating the operation to be performed on the string
print("Split the said string into 2 lists: words and separators:")
# Print the result of the test function applied to the modified string 's'
print(test(s))
Sample Output:
Original string: W3resource Python, Exercises. Split the said string into 2 lists: words and separators: [['W3resource', 'Python', 'Exercises.'], [' ', ', ']] Original string: The dance, held in the school gym, ended at midnight. Split the said string into 2 lists: words and separators: [['The', 'dance', 'held', 'in', 'the', 'school', 'gym', 'ended', 'at', 'midnight.'], [' ', ', ', ' ', ' ', ' ', ' ', ', ', ' ', ' ']] Original string: The colors in my studyroom are blue, green, and yellow. Split the said string into 2 lists: words and separators: [['The', 'colors', 'in', 'my', 'studyroom', 'are', 'blue', 'green', 'and', 'yellow.'], [' ', ' ', ' ', ' ', ' ', ' ', ', ', ', ', ' ']]
Flowchart:
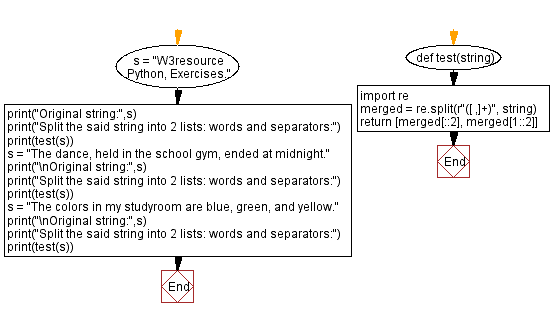
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: List of integers where the sum of the first i integers is i.
Next: List integers containing exactly three distinct values, such that no integer repeats twice consecutively.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics