Python: Find an increasing sequence consisting of the elements of the original list
Python Programming Puzzles: Exercise-85 with Solution
Write a Python program to find an increasing sequence consisting of the elements of the original list.
Input: [1, 3, 79, 10, 4, 2, 39] Output: [1, 2, 3, 4, 10, 39, 79] Input: [11, 31, 40, 68, 77, 93, 48, 1, 57] Output: [1, 11, 31, 40, 48, 57, 68, 77, 93] Input: [9, -2, 3, 4, -2, 0, 2, -3, 8, -1] Output: [-3, -2, -1, 0, 2, 3, 4, 8, 9]
Visual Presentation:
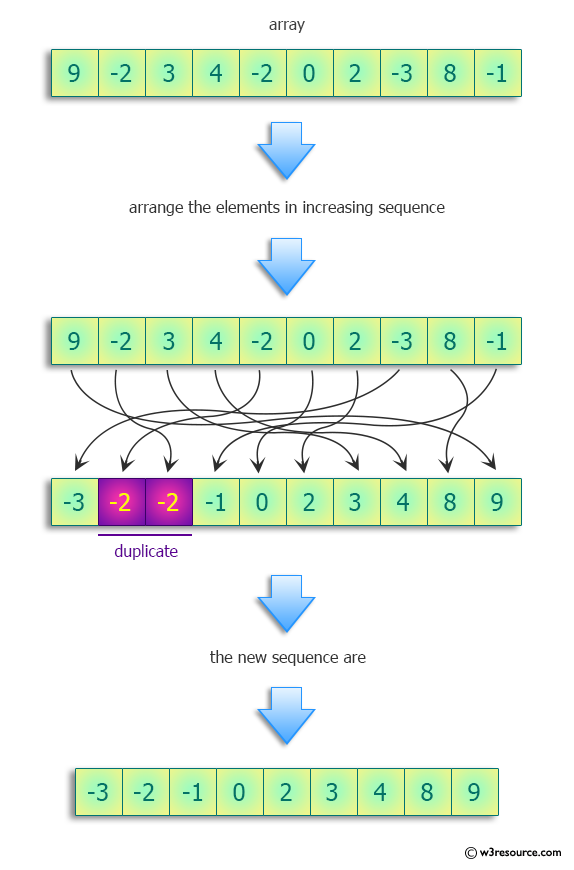
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Use the 'set' data structure to remove duplicates and then sort the unique elements
result = sorted(set(nums))
# Return the sorted and unique elements as the result
return result
# Example 1
nums1 = [1, 3, 79, 10, 4, 2, 39]
print("Original list of numbers:")
print(nums1)
print("Increasing sequence consisting of the elements of the said list:")
print(test(nums1))
# Example 2
nums2 = [11, 31, 40, 68, 77, 93, 48, 1, 57]
print("\nOriginal list of numbers:")
print(nums2)
print("Increasing sequence consisting of the elements of the said list:")
print(test(nums2))
# Example 3
nums3 = [9, -2, 3, 4, -2, 0, 2, -3, 8, -1]
print("\nOriginal list of numbers:")
print(nums3)
print("Increasing sequence consisting of the elements of the said list:")
print(test(nums3))
Sample Output:
Original list of numbers: [1, 3, 79, 10, 4, 2, 39] Increasing sequence consisting of the elements of the said list: [1, 2, 3, 4, 10, 39, 79] Original list of numbers: [11, 31, 40, 68, 77, 93, 48, 1, 57] Increasing sequence consisting of the elements of the said list: [1, 11, 31, 40, 48, 57, 68, 77, 93] Original list of numbers: [9, -2, 3, 4, -2, 0, 2, -3, 8, -1] Increasing sequence consisting of the elements of the said list: [-3, -2, -1, 0, 2, 3, 4, 8, 9]
Flowchart:
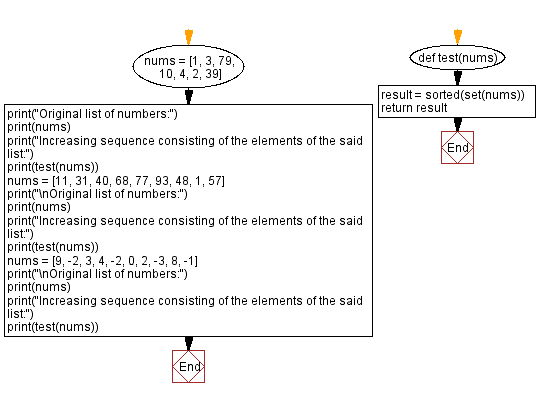
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the index of the matching parentheses for each character in a given string.
Next: Find the vowels from each of the original texts (y counts as a vowel at the end of the word).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-85.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics