Python: Find the vowels from each of the original texts (y counts as a vowel at the end of the word)
Python Programming Puzzles: Exercise-86 with Solution
Write a Python program to find the vowels from each of the original texts (y counts as a vowel at the end of the word) from a given list of strings.
Input: ['w3resource', 'Python', 'Java', 'C++'] Output: ['eoue', 'o', 'aa', ''] Input: ['ably', 'abruptly', 'abecedary', 'apparently', 'acknowledgedly'] Output: ['ay', 'auy', 'aeeay', 'aaey', 'aoeey']
Visual Presentation:
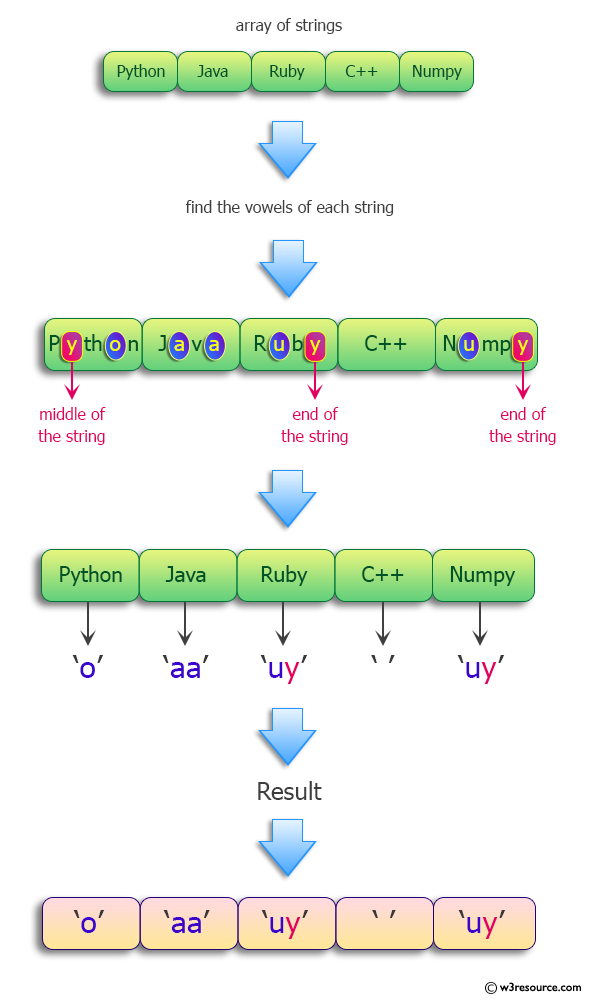
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of strings 'strs' as input
def test(strs):
# Use a list comprehension to iterate over each text in 'strs'
return [
"".join(c for c in text if c.lower() in "aeiou") + (text[-1] if text[-1].lower() == "y" else "")
for text in strs
]
# Example 1
strs1 = ["w3resource", "Python", "Java", "C++"]
print("Original List of strings:", strs1)
print("Vowels from each of the original texts (y counts as a vowel at the end of the word:")
print(test(strs1))
# Example 2
strs2 = ["ably", "abruptly", "abecedary", "apparently", "acknowledgedly"]
print("\nOriginal List of strings:", strs2)
print("Positions of all uppercase vowels (not counting Y) in even indices:")
print(test(strs2))
Sample Output:
Original List of strings: ['w3resource', 'Python', 'Java', 'C++'] Vowels from each of the original texts (y counts as a vowel at the end of the word: ['eoue', 'o', 'aa', ''] Original List of strings: ['ably', 'abruptly', 'abecedary', 'apparently', 'acknowledgedly'] Positions of all uppercase vowels (not counting Y) in even indices: ['ay', 'auy', 'aeeay', 'aaey', 'aoeey']
Flowchart:
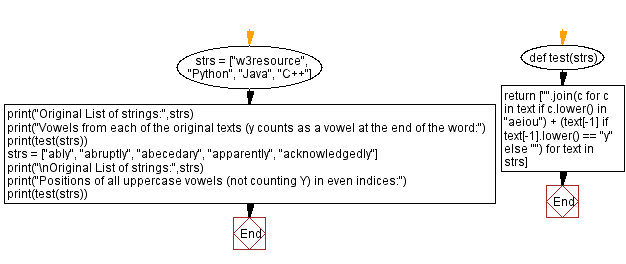
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find an increasing sequence consisting of the elements of the original list.
Next: Find a valid substring of s that contains matching brackets, at least one of which is nested.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-86.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics