Python: Single digits in numbers sorted backwards and converted to English words
Python Programming Puzzles: Exercise-96 with Solution
Write a Python program to get the single digits in numbers sorted backwards and converted into English words.
Input: [1, 3, 4, 5, 11] Output: ['five', 'four', 'three', 'one'] Input: [27, 3, 8, 5, 1, 31] Output: ['eight', 'five', 'three', 'one']
Visual Presentation:
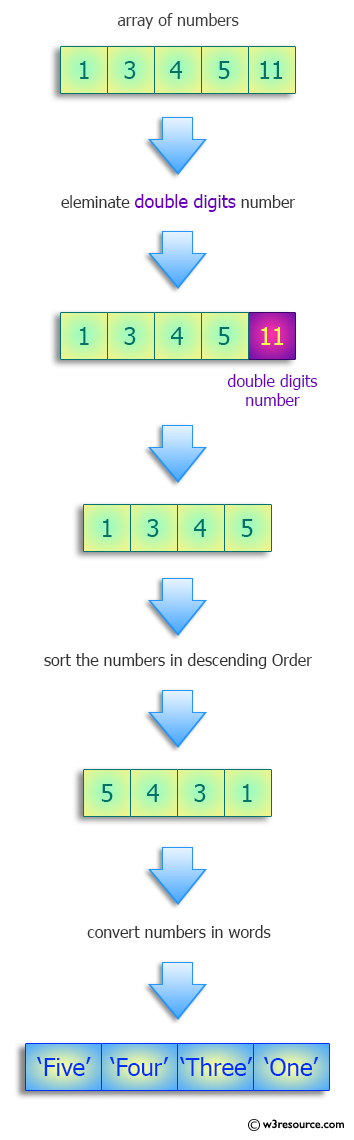
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Function to convert single-digit numbers in a list to English words,
# sort them backwards, and return the result
def test(nums):
# Dictionary mapping English words to their corresponding digits
digits = {
"zero": None,
"one": 1,
"two": 2,
"three": 3,
"four": 4,
"five": 5,
"six": 6,
"seven": 7,
"eight": 8,
"nine": 9
}
# Create a reverse dictionary mapping digits to their corresponding English words
digits_backwards = {digits[k]: k for k in digits}
# Convert the original dictionary values to a list
digits = [digits[s] for s in digits]
# Extract single-digit numbers from the input list and convert them to English words
li = [digits[n] for n in nums if n in digits]
# Return the sorted list of English words corresponding to the digits
return [digits_backwards[n] for n in sorted(li, reverse=True)]
# Test cases with different lists of numbers
nums = [1, 3, 4, 5, 11]
print("Original list of numbers:")
print(nums)
print("Return the single digits in nums sorted backwards and converted to English words:")
print(test(nums))
nums = [27, 3, 8, 5, 1, 31]
print("\nOriginal list of numbers:")
print(nums)
print("Return the single digits in nums sorted backwards and converted to English words:")
print(test(nums))
Sample Output:
Original list of numbers: [1, 3, 4, 5, 11] Return the single digits in nums sorted backwards and converted to English words: ['five', 'four', 'three', 'one'] Original list of numbers: [27, 3, 8, 5, 1, 31] Return the single digits in nums sorted backwards and converted to English words: ['eight', 'five', 'three', 'one']
Flowchart:
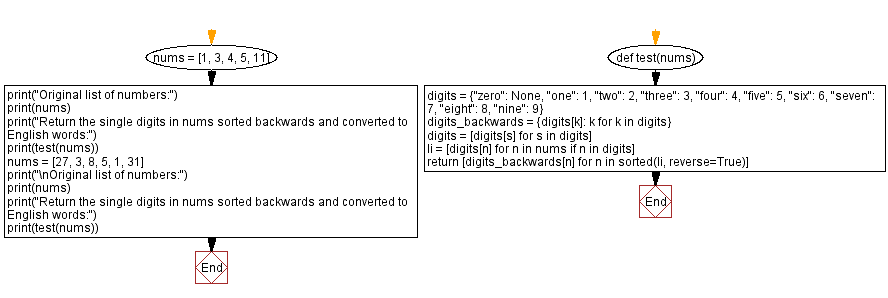
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find a palindrome of a given length containing a given string.
Next: Strange sort of list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/puzzles/python-programming-puzzles-96.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics