Python PyQt text display application
Python PyQt Basic: Exercise-5 with Solution
Write a Python program using PyQt5 that creates an application with a QPushButton and a QLabel. Whenever the button is clicked, the label's text should change.
Note:
The QPushButton widget provides a command button.
The QLabel widget provides a text or image display.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QLabel Class: The QLabel widget provides a text or image display.
QVBoxLayout Class: This class is used to construct vertical box layout objects.
QWidget: The QWidget class is the base class of all user interface objects.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QLabel, QVBoxLayout, QWidget
class TextChangeApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Text Change Application")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QLabel to display text
self.label = QLabel("Python Exercises, click the button to get new exercises.")
# Create a QPushButton
self.button = QPushButton("Change Text")
# Create a vertical layout
layout = QVBoxLayout()
# Add widgets to the layout
layout.addWidget(self.label)
layout.addWidget(self.button)
# Set the layout for the central widget
central_widget.setLayout(layout)
# Connect the button click event to the change_text function
self.button.clicked.connect(self.change_text)
def change_text(self):
# Change the text of the label when the button is clicked
new_text = "Java Exercises!"
self.label.setText(new_text)
def main():
# Create a PyQt application
app = QApplication(sys.argv)
# Create an instance of the TextChangeApp class
window = TextChangeApp()
# Show the window
window.show()
# Run the application's event loop
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a custom "TextChangeApp" class that inherits from "QMainWindow."
- Inside the class, create the window and widgets (QLabel and QPushButton) as well as set their properties and layout.
- Connect the button's click event to the change_text method, which changes the QLabel text when the button is clicked.
- In the main function, we create an instance of 'TextChangeApp', show the window, and start the application's event loop.
Output:
Flowchart:
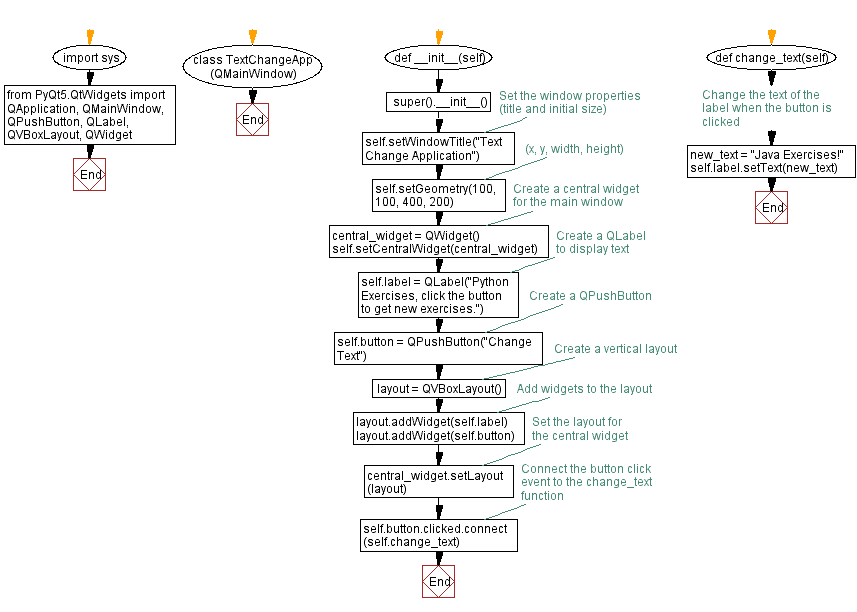
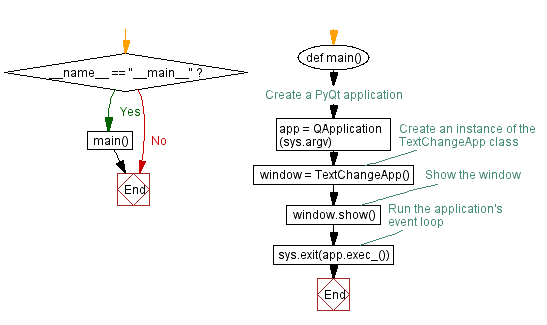
Python Code Editor:
Previous: Creating a text display application with PyQt.
Next: Creating a custom widget with PyQt.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pyqt/python-pyqt-basic-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics