Python PyQt button click example
Python PyQt Connecting Signals to Slots: Exercise-1 with Solution
Write a Python program that creates a PyQt application with a push button. Display a message when the push button is clicked.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QPushButton Class: The QPushButton widget provides a command button.
QMessageBox Class: The QMessageBox class provides a modal dialog for informing the user or for asking the user a question and receiving an answer.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QMessageBox
# Define a slot function to display a message when the button is clicked
def show_message():
message_box = QMessageBox()
message_box.setWindowTitle("Message")
message_box.setText("Button clicked!")
message_box.exec_()
def main():
app = QApplication(sys.argv)
# Create the main window
window = QWidget()
window.setWindowTitle("PyQt Button Click Example")
window.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a push button
button = QPushButton("Click me!", window)
button.setGeometry(150, 80, 100, 40) # (x, y, width, height)
# Connect the button's clicked signal to the show_message slot
button.clicked.connect(show_message)
# Show the main window
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Define a slot function named "show_message()" that creates a QMessageBox to display a message when called.
- In the main function, create the PyQt application and the main window.
- Create a "QPushButton" widget labeled "Click me!" and set its geometry (position and size) within the window.
- Connect the clicked signal of the button to the "show_message()" slot using the clicked.connect() method.
- Show the main window using window.show().
- Finally, we start the PyQt application's event loop using app.exec_().
Output:
Flowchart:
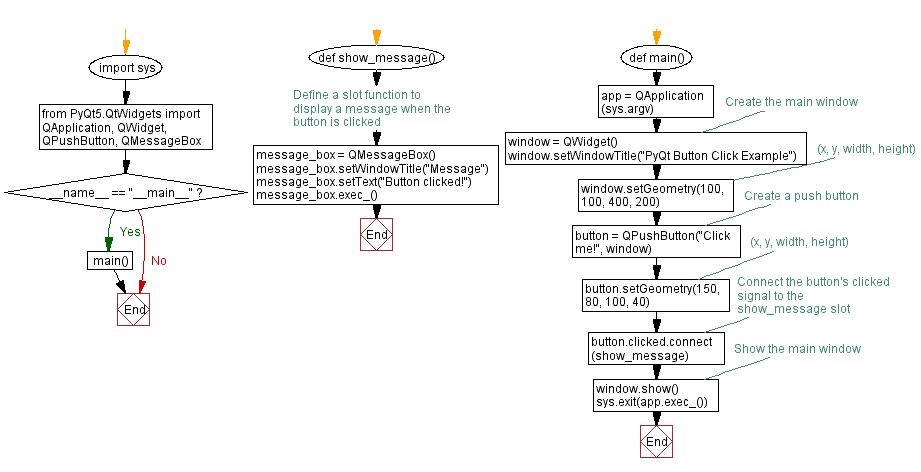
Python Code Editor:
Previous: Python PyQt Connecting signals to Slots Home.
Next: Python PyQt button actions example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/pyqt/python-pyqt-connecting-signals-to-slots-exercise-1.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics