Python PyQt radio button example
Write a Python program that creates a PyQt application with a radio button group. When you select a radio button it should immediately show you which one you picked.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QRadioButton Class: The QRadioButton widget provides a radio button with a text label.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QLabel Class: The QLabel widget provides a text or image display.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QRadioButton, QVBoxLayout, QLabel
class RadioButtonApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Radio Button Example")
self.setGeometry(100, 100, 300, 200) # (x, y, width, height)
layout = QVBoxLayout()
# Create radio buttons for options
self.option1_radio = QRadioButton("Python", self)
self.option2_radio = QRadioButton("Java", self)
self.option3_radio = QRadioButton("C++", self)
layout.addWidget(self.option1_radio)
layout.addWidget(self.option2_radio)
layout.addWidget(self.option3_radio)
# Create a label to display the selected option
self.selected_option_label = QLabel("Selected Option: None", self)
layout.addWidget(self.selected_option_label)
# Connect the radio buttons' toggled signal to a slot that updates the label
self.option1_radio.toggled.connect(lambda: self.update_selected_option("Python"))
self.option2_radio.toggled.connect(lambda: self.update_selected_option("Java"))
self.option3_radio.toggled.connect(lambda: self.update_selected_option("C++"))
self.setLayout(layout)
def update_selected_option(self, option):
if option:
self.selected_option_label.setText(f"Selected Option: {option}")
def main():
app = QApplication(sys.argv)
window = RadioButtonApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QWidget" class named "RadioButtonApp" that builds a radio button group with options.
- Design a layout with three radio buttons for options ('Python', 'Java', 'C++').
- Create a "QLabel" (self.selected_option_label) to display the selected option.
- Connect the toggle signal of each radio button to a slot (self.update_selected_option) that updates the label with the selected option.
- In the main function, we create the PyQt application, instantiate the "RadioButtonApp" class, and show the main window.
Output:
Flowchart:
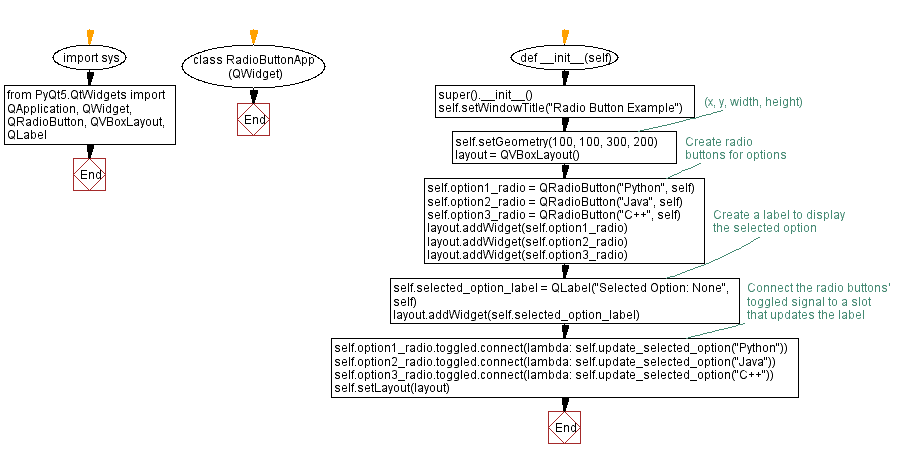
Go to:
Previous: Python PyQt simple calculator.
Next: Python PyQt close window confirmation.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.