Python PyQt close window confirmation
Write a Python program that creates a window for an application. When you try to close the window (like clicking the X button), it should check if you're sure you want to close the application and ask for confirmation. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QMessageBox Class: The QMessageBox class provides a modal dialog for informing the user or for asking the user a question and receiving an answer.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QMessageBox
class MyMainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Close Confirmation")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
def closeEvent(self, event):
# Ask for confirmation before closing
confirmation = QMessageBox.question(self, "Confirmation", "Are you sure you want to close the application?", QMessageBox.Yes | QMessageBox.No)
if confirmation == QMessageBox.Yes:
event.accept() # Close the app
else:
event.ignore() # Don't close the app
def main():
app = QApplication(sys.argv)
window = MyMainWindow()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QMainWindow" class named "MyMainWindow" that sets up the main application window.
- Override the closeEvent method to handle window close events.
- Inside the "closeEvent()" method, it displays a confirmation dialog asking if you're sure you want to close the application.
- If you click "Yes" in the confirmation dialog, it accepts the close event, and the application closes. If you click "No," it ignores the close event, and the application stays open.
- In the main function, we create the PyQt application, instantiate the "MyMainWindow" class, and show the main window.
Output:
Flowchart:
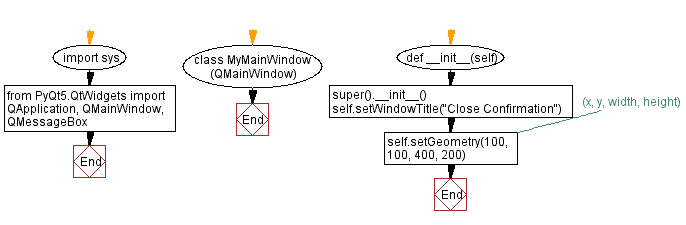
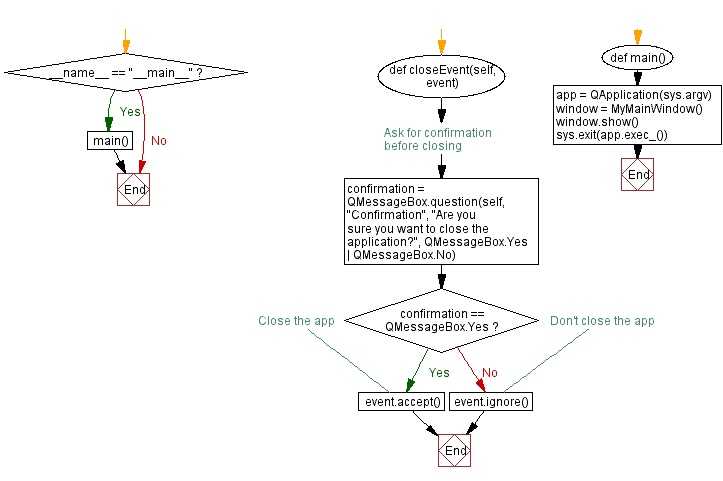
Go to:
Previous: Python PyQt radio button example.
Next: Python PyQt time display.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.