Python PyQt menu example
Write a Python program that creates an application with a menu. When we choose menu items, a specific message appears.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QMenu Class: The QMenu class provides a menu widget for use in menu bars, context menus, and other popup menus.
QAction Class: The QAction class provides an abstraction for user commands that can be added to different user interface components.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QMenu, QAction
class MenuApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Menu Example")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a menu bar
menu_bar = self.menuBar()
# Create a menu called "File"
file_menu = QMenu("File", self)
menu_bar.addMenu(file_menu)
# Create actions for menu items
open_action = QAction("Open", self)
save_action = QAction("Save", self)
exit_action = QAction("Exit", self)
# Connect the menu actions to functions that do something
open_action.triggered.connect(self.open_file)
save_action.triggered.connect(self.save_file)
exit_action.triggered.connect(self.close)
# Add the actions to the "File" menu
file_menu.addAction(open_action)
file_menu.addAction(save_action)
file_menu.addSeparator() # Adds a separator line in the menu
file_menu.addAction(exit_action)
def open_file(self):
print("Open file menu item selected")
def save_file(self):
print("Save file menu item selected")
def main():
app = QApplication(sys.argv)
window = MenuApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QMainWindow" class named "MenuApp" that builds the main application window.
- Set up a menu bar and created a menu called "File."
- Inside the "File" menu, we create actions for menu items like "Open," "Save," and "Exit."
- Connect each menu action to a function (self.open_file, self.save_file, self.close) that does something when the menu items are selected.
- In the main() function, we create the PyQt application, instantiate the "MenuApp" class, and show the main window.
Output:
Open file menu item selected Save file menu item selected![]()
Flowchart:
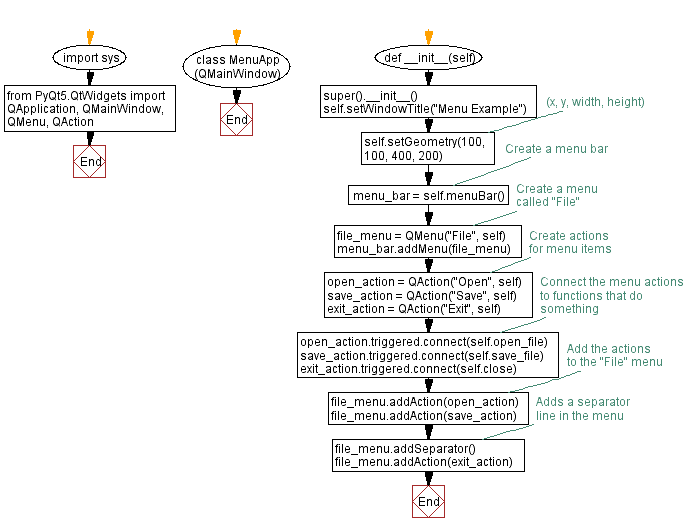
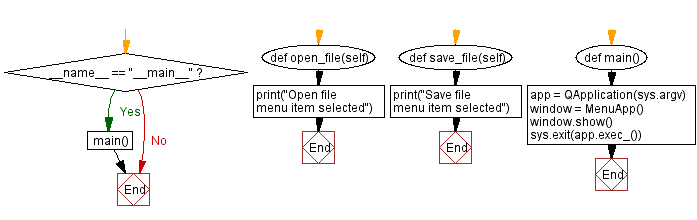
Python Code Editor:
Previous: Python PyQt checkbox example.
Next: Python PyQt keyboard shortcut example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.