Python PyQt keyboard shortcut example
Write a Python program that makes an application listen to keyboard shortcuts, like pressing a specific key combination. When you press that key combination, a message appears. Use the PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
QKeySequence Class: The QKeySequence class encapsulates a key sequence as used by shortcuts.
Qt module: PyQt5 is a set of Python bindings for the Qt application framework. It allows us to use Qt, a popular C++ framework, to create graphical user interfaces (GUIs) in Python.
QShortcut Class: The QShortcut class is used to create keyboard shortcuts.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel
from PyQt5.QtGui import QKeySequence
from PyQt5.QtCore import Qt
from PyQt5.QtWidgets import QShortcut
class ShortcutApp(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Shortcut Example")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a label to display messages
self.message_label = QLabel("Press Ctrl+M to show a message", self)
self.message_label.setAlignment(Qt.AlignmentFlag.AlignCenter)
self.setCentralWidget(self.message_label)
# Create a keyboard shortcut Ctrl+M
shortcut = QKeySequence(Qt.CTRL + Qt.Key_M)
self.shortcut = QShortcut(shortcut, self)
self.shortcut.activated.connect(self.show_message)
def show_message(self):
# Display a message when the shortcut is activated
self.message_label.setText("You pressed Ctrl+M!")
def main():
app = QApplication(sys.argv)
window = ShortcutApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QMainWindow" class named "ShortcutApp" that builds the main application window.
- "QLabel" (self.message_label) displays messages inside the window.
- Set up a keyboard shortcut (Ctrl+M) using QKeySequence.
- Connect the shortcut's activated signal to a slot function (self.show_message) that displays a message.
- Create a QShortcut object named self.shortcut and set its shortcut to Ctrl+S.
- In the "show_message()" slot function, we change the label's text to "You pressed Ctrl+M!" when the shortcut is activated.
- In the main function, we create the PyQt application, instantiate the ShortcutApp class, and show the main window.
Output:
Flowchart:
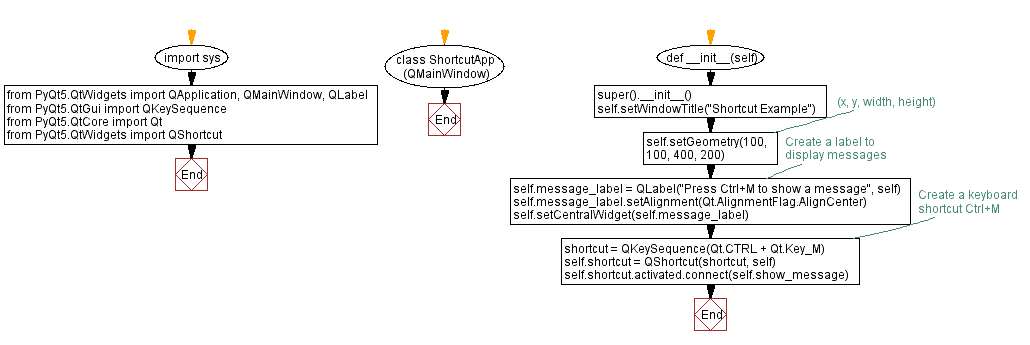
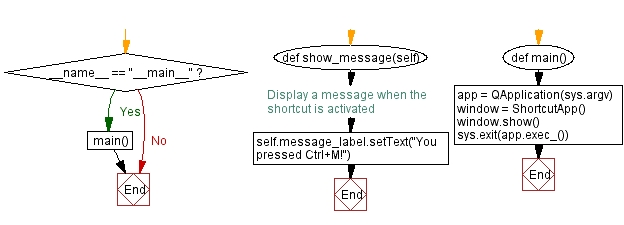
Python Code Editor:
Previous: Python PyQt menu example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics