Python PyQt text updater application
Write a Python program that creates a one-line text editor where you can type text. Whenever you type something in that box, it should immediately show up somewhere else on the screen, like a label. Use PyQt module.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget Class: The QWidget class is the base class of all user interface objects.
QLineEdit Class: The QLineEdit widget is a one-line text editor.
QLabel Class: The QLabel widget provides a text or image display.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLineEdit, QLabel, QVBoxLayout
class TextUpdaterApp(QWidget):
def __init__(self):
super().__init__()
self.setWindowTitle("Text Updater App")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
layout = QVBoxLayout()
# Create a QLineEdit widget
self.line_edit = QLineEdit(self)
layout.addWidget(self.line_edit)
# Create a QLabel widget
self.label = QLabel("Text Entered: ", self)
layout.addWidget(self.label)
# Connect the textChanged signal to the update_label slot
self.line_edit.textChanged.connect(self.update_label)
self.setLayout(layout)
# Slot function to update the label text
def update_label(self):
text = self.line_edit.text()
self.label.setText("Text Entered: " + text)
def main():
app = QApplication(sys.argv)
window = TextUpdaterApp()
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a custom "QWidget" class named "TextUpdaterApp" that inherits from QWidget.
- Inside the TextUpdaterApp class, we create a QLineEdit widget (self.line_edit) and a QLabel widget (self.label). Both widgets are added to a QVBoxLayout for layout management.
- Connect the textChanged signal of the "QLineEdit" to the update_label slot function using self.line_edit.textChanged.connect(self.update_label). This means that whenever the text in the line edit changes, the "update_label()" function will be called to update the label text.
- The "update_label()" slot function retrieves the text from the "QLineEdit" using self.line_edit.text() and updates the QLabel's text to display "Text Entered: " followed by the entered text.
- In the main function, we create the PyQt application, instantiate the TextUpdaterApp class, and show the main window.
Output:
Flowchart:
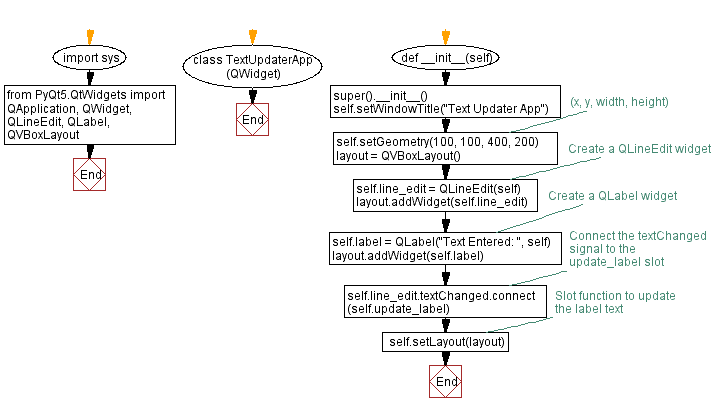
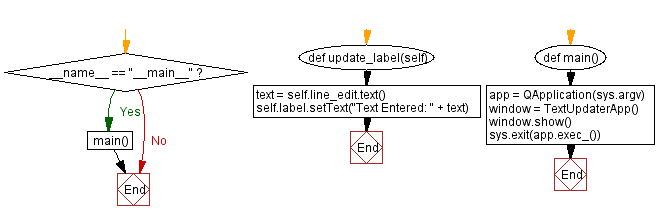
Python Code Editor:
Previous: Python PyQt button actions example.
Next: Python PyQt slider application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics